统计英文文本词频并可视化输出前十词频
时间: 2024-11-27 08:22:06 浏览: 0
统计英文文本词频并可视化输出前十词频的过程通常涉及以下几个步骤:
1. **数据预处理**:首先需要对文本进行清洗,去除标点符号、数字和其他非字母字符,并将所有单词转换为小写形式。可以使用Python的`nltk`或`spaCy`库进行这一步。
```python
import re
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
def preprocess_text(text):
text = re.sub(r'\W+', ' ', text).lower() # 删除非字母字符并转为小写
stop_words = set(stopwords.words('english')) # 加载英语停用词
words = [word for word in word_tokenize(text) if word not in stop_words]
return words
```
2. **词频计算**:然后使用`collections.Counter`来计算每个单词的频率。
```python
from collections import Counter
text = "..." # 输入你的英文文本
preprocessed_words = preprocess_text(text)
word_counts = Counter(preprocessed_words)
```
3. **排序和取前十个高频词**:
```python
top_10_words = word_counts.most_common(10)
```
4. **可视化结果**:最后,你可以使用matplotlib或者seaborn等库将结果可视化成条形图或者饼图。
```python
import matplotlib.pyplot as plt
def visualize_word_freq(word_list, freq_list):
plt.bar(range(len(freq_list)), freq_list)
plt.xticks(range(len(freq_list)), word_list)
plt.xlabel("Words")
plt.ylabel("Frequency")
plt.title("Top 10 Most Frequent Words")
plt.show()
visualize_word_freq([x[0] for x in top_10_words], [x[1] for x in top_10_words])
```
阅读全文
相关推荐
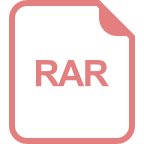
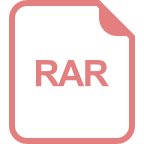
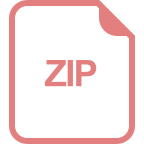
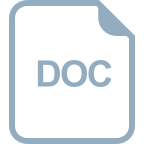
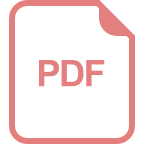
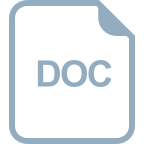
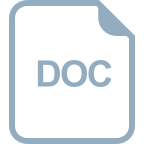
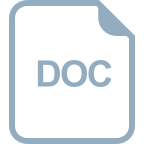
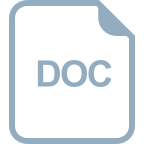









