python中str((int)value) 这个我该如何修改
时间: 2024-09-24 15:16:29 浏览: 47
在Python中,如果你想将整数 `value` 转换为字符串并存储在一个变量里,可以使用内置函数 `str()` 直接应用到整数值上。例如:
```python
value = 123
str_value = str(value)
```
这里 `str_value` 就会是一个包含数字 "123" 的字符串。
如果你的 `value` 已经是字符串类型,并且想要检查或转换它是否为整数,可以用 `isinstance()` 函数来检查:
```python
value = "123"
if isinstance(value, int):
str_value = str(value)
else:
print(f"{value} is not an integer, it's a string.")
```
如果你想确保值始终被转换成字符串,无论它是哪种类型的数值(整数、浮点数等),可以直接这样做:
```python
value = "123" or 456
str_value = str(int(value) if isinstance(value, (int, float)) else value)
```
这里如果 `value` 是数字,则先强制转换为整数再转成字符串;如果不是数字,则保持原样。
相关问题
python str转int
要将Python中的字符串转换为整数,您可以使用内置的int()函数。例如:
```python
str_num = "123"
int_num = int(str_num)
print(int_num) # 输出: 123
```
请注意,如果字符串包含非数字字符,则会引发ValueError异常。如果您希望在出现错误时提供默认值,可以使用try-except块来捕获异常。例如:
```python
str_num = "123abc"
default_value = 0 # 默认值为0
try:
int_num = int(str_num)
except ValueError:
int_num = default_value
print(int_num) # 输出: 0
```
希望这可以帮助到您!如果还有其他问题,请随时提问。
把这串代码转换为python语法def int_to_str(n): if n < 10: return str(n) else: return int_to_str(n // 10) + str(n % 10)
Null is a term used in computer programming and databases to represent an absence of a value or a variable that has not been assigned a value. It is often represented as a zero or a blank space, but it is not the same as zero or an empty string. In programming languages such as Java and Python, null is used to indicate the absence of an object, whereas in SQL databases, it is used to represent a missing or unknown value.
阅读全文
相关推荐
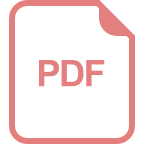
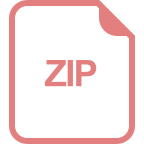
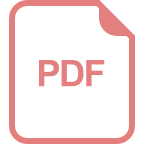
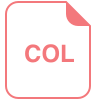












