C#多设备多协议同时工作,串口,scoket系统代码
时间: 2024-11-22 17:34:22 浏览: 8
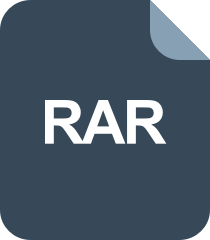
C#串口通讯和socket通讯demo
在C#中,如果你需要让程序支持多种设备通过不同的通信协议(如串口、Socket等)同时工作,你需要做的是:
1. **引用相关的库**:
- 对于串口通信,通常使用`System.IO.Ports`命名空间,包含SerialPort类,用于处理串行端口(COM端口)的操作。
- 对于网络通信(包括TCP和UDP),可以使用System.Net.Sockets命名空间下的Socket类或TcpClient/TcpListener/SocketServer等。
```csharp
using System.IO.Ports;
using System.Net.Sockets;
```
2. **创建通信接口**:
创建一个抽象基类或者接口,定义公共的方法签名,比如发送数据、接收数据等。这样,无论是串口还是Socket,都需实现这个接口。
```csharp
public interface ICommunicationDevice
{
void Send(string data);
string Receive();
}
```
3. **具体实现**:
- 对于`SerialPort`,你可以创建一个`SerialPortAdapter`类,继承自`ICommunicationDevice`,并在其中封装SerialPort的功能。
- 对于`Socket`,可以创建`SocketAdapter`类,同样遵循接口,使用Socket的实例来实现连接和数据传输。
```csharp
public class SerialPortAdapter : ICommunicationDevice
{
public void Send(string data)
{
// 使用SerialPort.SendData方法...
}
public string Receive()
{
// 读取SerialPort的输入...
}
}
public class SocketAdapter : ICommunicationDevice
{
private TcpClient client;
public void Connect(string host, int port)
{
client = new TcpClient(host, port);
}
public void Send(string data)
{
// 发送到Socket客户端...
}
public string Receive()
{
// 从Socket接收数据...
}
}
```
4. **多设备多协议管理**:
最后,在主程序里,你可以创建一个设备池或者策略模式,根据实际需求动态选择合适的通信适配器。
```csharp
List<ICommunicationDevice> devices = new List<ICommunicationDevice>();
devices.Add(new SerialPortAdapter());
// 或者添加SocketAdapter实例
foreach (var device in devices)
{
if (device is SerialPortAdapter serialPort)
{
serialPort.Open();
}
else if (device is SocketAdapter socket)
{
socket.Connect("remote_host", remote_port);
}
// 根据设备类型进行操作
device.Send(data);
var response = device.Receive();
}
阅读全文
相关推荐
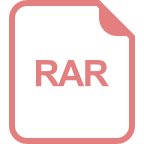
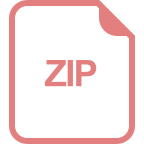



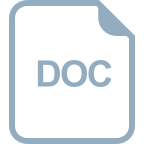
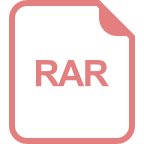
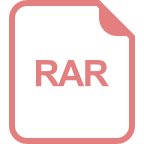
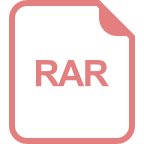
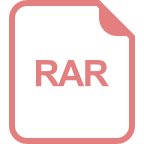
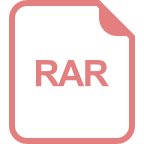
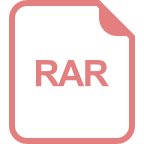
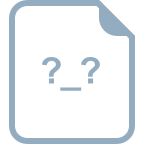
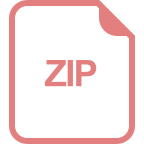
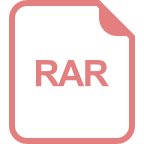
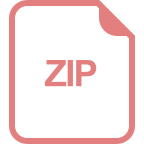
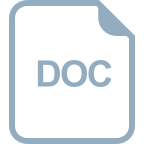

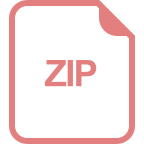