pycharm文字代码
时间: 2023-10-11 22:11:47 浏览: 88
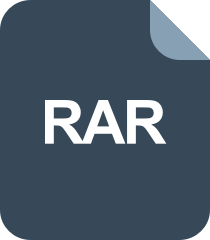
Python编程 3-8-2. 如何在 PyCharm 控制台中输出彩色文字和背景.rar
当然,我可以帮你提供一些PyCharm中的文字代码。以下是一个简单的示例:
```python
print("Hello, World!")
```
这段代码会在控制台打印出"Hello, World!"。你可以在PyCharm中创建一个新的Python文件,将该代码复制粘贴进去,然后点击运行按钮来执行它。
如果你有其他关于PyCharm的问题,或者需要更复杂的代码示例,请随时告诉我!
阅读全文
相关推荐
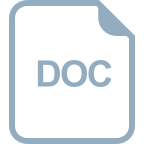
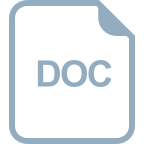


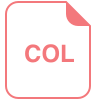








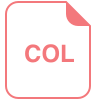
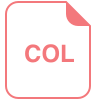
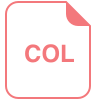

