python3 提取发票信息
时间: 2023-06-28 10:01:54 浏览: 163
### 回答1:
Python3 可以使用一些库来提取发票信息,其中最常用的是 pytesseract以及 OpenCV。
首先,我们需要安装 pytesseract 和 OpenCV 的库。可以使用以下命令进行安装:
```
pip install pytesseract opencv-python
```
安装完成后,我们可以使用 pytesseract 的 OCR 功能来识别图片中的文本,并通过 OpenCV 进行图片处理。
```python
import cv2
import pytesseract
# 读取图片
image = cv2.imread('invoice.jpg')
# 转为灰度图像
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 使用二值化处理,提高文字识别的准确度
_, threshold_image = cv2.threshold(gray_image, 0, 255, cv2.THRESH_BINARY | cv2.THRESH_OTSU)
# 通过 pytesseract 识别文本
text = pytesseract.image_to_string(threshold_image, lang='eng')
# 输出识别结果
print(text)
```
以上代码将读取名为 "invoice.jpg" 的图片,将其转换为灰度图像,并使用二值化处理。然后,使用 pytesseract 的 `image_to_string` 方法识别图像中的文本,并将结果输出。
需要注意的是,提取发票信息可能涉及到图片预处理、文本分析等复杂的操作。以上代码仅提供了一个基本的示例,具体的提取过程可能需要根据实际情况进行调整和优化。
### 回答2:
要提取发票信息,可以使用Python3中的相关库和技术来完成。以下是一个简单的步骤:
1. 导入必要的库:首先需要导入Python中的一些库,例如OpenCV、Tesseract等。这些库可以用来处理图像和文字识别。
2. 加载发票图像:使用OpenCV库加载发票的图像文件,确保图像清晰且文字清晰可辨。
3. 文字识别:使用Tesseract库进行文字识别,可以将发票图像中的文本提取出来。可以使用以下命令将图像转化为可识别的文本:
```
import pytesseract
text = pytesseract.image_to_string(image, lang='chi_sim')
```
这里的`image`是发票图像的OpenCV对象,`lang='chi_sim'`表示使用中文字符集。
4. 提取所需信息:根据所需的发票信息,利用字符串处理技术、正则表达式等方法提取出关键字或特定格式的文本。例如,要提取发票号码,可以使用正则表达式`r'发票号码:(\w+)'`来匹配发票号码的文本。根据实际发票的布局和格式,可以编写相应的提取规则。
5. 输出提取结果:最后,将提取到的发票信息进行输出,可以选择以文本、JSON、CSV等格式进行输出,以便进一步处理或存储。
总之,通过使用适当的库和技术,结合图像处理和文字识别,可以完成Python3中的发票信息提取任务。具体的实现细节可以根据实际需求进行调整。
相关推荐
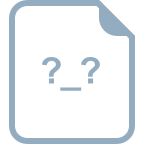
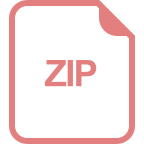














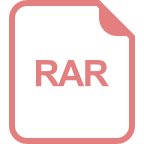