matplotlib绘制折线图,中间某段时间基本无数据,如何压缩?
时间: 2023-08-11 16:14:17 浏览: 42
如果中间某段时间基本无数据,可以使用matplotlib中的`set_xlim()`方法来压缩该时间段。具体操作如下:
1. 找到需要压缩的时间段的起始和终止时间,可以通过查看数据集或者手动指定。
2. 计算该时间段的起始和终止时间对应的横坐标值。
3. 使用`set_xlim()`方法设置横坐标范围,即将起始和终止时间对应的横坐标值作为参数传入即可。
下面是一个示例代码:
```python
import matplotlib.pyplot as plt
import numpy as np
# 生成数据
x = np.arange(0, 10, 0.1)
y = np.sin(x)
# 将第3秒到第7秒的数据进行压缩
start_time = 3
end_time = 7
start_index = int(start_time * 10)
end_index = int(end_time * 10)
x_min = x[start_index]
x_max = x[end_index]
# 绘制折线图
plt.plot(x, y)
plt.xlim(x_min, x_max) # 设置横坐标范围
plt.show()
```
执行以上代码后,将会绘制出一张sin函数的折线图,其中第3秒到第7秒的数据被压缩在一起。
相关问题
python折线图绘制某段变色
要在Python中绘制某段变色的折线图,可以使用Matplotlib库来实现。首先,您需要准备好要绘制的数据。然后,使用Matplotlib的plot函数来绘制折线图,并使用fill_between函数来填充某段变色。下面是一个简单的示例代码:
import matplotlib.pyplot as plt
# 准备数据
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# 绘制折线图
plt.plot(x, y)
# 指定要变色的段落范围
start = 2
end = 4
# 使用fill_between函数填充某段变色
plt.fill_between(x[start-1:end], y[start-1:end], color='yellow')
# 显示图形
plt.show()
在这个示例中,我们首先准备了x和y坐标的数据。然后,使用plot函数绘制了折线图。接下来,指定了要变色的段落范围,即start和end。最后,使用fill_between函数来填充某段变色的区域,这里我们选择的颜色是黄色。最后调用show函数显示图形。
您可以根据您的具体需求修改示例代码中的数据和颜色,以实现您想要的效果。
可是我只想将全部数据绘制,中间某段时间的数据不显示,因为我在绘制时间序列折线图
明白了,你可以考虑使用`fill_between`函数来实现这个效果。该函数可以在折线图上绘制一个填充区域,从而达到隐藏某个时间段数据的效果。
下面是一个示例代码:
```python
import matplotlib.pyplot as plt
import numpy as np
# 生成数据
x = np.arange(0, 10, 0.1)
y = np.sin(x)
# 隐藏第3秒到第7秒的数据
start_time = 3
end_time = 7
start_index = int(start_time * 10)
end_index = int(end_time * 10)
# 绘制折线图
plt.plot(x, y)
# 绘制填充区域
plt.fill_between(x[start_index:end_index], y[start_index:end_index], np.min(y), color='white')
plt.show()
```
执行以上代码后,将会绘制出一张sin函数的折线图,其中第3秒到第7秒的数据被隐藏了起来。注意,这里使用`fill_between`函数绘制的填充区域的颜色设置为白色,是为了将该区域的颜色与背景色保持一致,从而达到隐藏数据的效果。如果需要调整填充区域的颜色,可以将`color`参数设置为其他颜色。
相关推荐
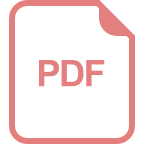
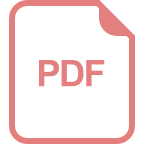
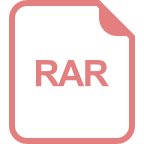













