用python创建一个类来保存数据矩阵,类的属性是行数,列数和保留矩阵元素的列表。定义一个函数在给定行数和烈数的情况下创建一个空矩阵。满足以下要求:1将两个矩阵相加(两个矩阵有相同的维数)2.将两个矩阵相乘。要求:输入一个矩阵,分别返回以上两个个要求的结果。
时间: 2024-09-13 21:15:36 浏览: 34
你可以创建一个名为`Matrix`的Python类,它包含三个属性:`rows`、`cols`和`elements`,用于表示矩阵的行数、列数以及矩阵元素的列表。下面是一个基本的实现:
```python
class Matrix:
def __init__(self, rows, cols):
self.rows = rows
self.cols = cols
self.elements = []
def add_matrix(self, other):
if self.rows != other.rows or self.cols != other.cols:
raise ValueError("Matrices must have the same dimensions to be added.")
result = [[0] * self.cols for _ in range(self.rows)]
for i in range(self.rows):
for j in range(self.cols):
result[i][j] = self.elements[i * self.cols + j] + other.elements[i * other.cols + j]
return result
def multiply_matrix(self, other):
if self.cols != other.rows:
raise ValueError("The number of columns in the first matrix must equal the number of rows in the second matrix to multiply.")
result = [[0] * other.cols for _ in range(self.rows)]
for i in range(self.rows):
for j in range(other.cols):
for k in range(self.cols):
result[i][j] += self.elements[i * self.cols + k] * other.elements[k * other.cols + j]
return result
def create_empty_matrix(self, rows, cols):
self.rows = rows
self.cols = cols
self.elements = [[0] * cols for _ in range(rows)]
return self
def print_matrix(self):
for row in self.elements:
print(row)
```
现在你可以实例化这个类并操作矩阵了:
```python
# 创建一个2x2的零矩阵
matrix1 = Matrix(2, 2).create_empty_matrix(2, 2)
# 添加两个矩阵
matrix2 = Matrix(2, 2).create_empty_matrix(2, 2)
matrix2.elements = [[1, 2], [3, 4]]
sum_matrix = matrix1.add_matrix(matrix2)
# 计算并打印乘积
matrix3 = Matrix(2, 2).create_empty_matrix(2, 2)
matrix3.elements = [[5, 6], [7, 8]]
product_matrix = matrix1.multiply_matrix(matrix3)
print("Sum:")
matrix1.print_matrix()
print("\nProduct:")
matrix3.print_matrix()
```
阅读全文
相关推荐
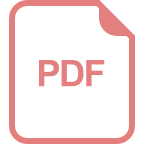
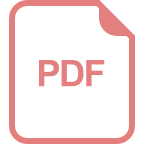
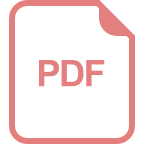




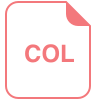










