用C + +与easyX写一个五子棋
时间: 2023-07-04 09:04:58 浏览: 89
好的,下面是一个简单的五子棋代码,使用 C++ 和 EasyX 图形库。
```cpp
#include <iostream>
#include <graphics.h>
using namespace std;
const int kBoardSize = 15; // 棋盘大小
const int kGridSize = 40; // 网格大小
const int kRadius = 16; // 棋子半径
const int kMargin = 30; // 边距
// 棋盘坐标转换为像素坐标
int CoordinateToPixel(int coord) {
return kMargin + coord * kGridSize;
}
// 绘制棋盘
void DrawBoard() {
setbkcolor(RGB(240, 190, 60)); // 设置背景颜色
cleardevice(); // 清空画布
// 绘制横线和竖线
for (int i = 0; i < kBoardSize; ++i) {
line(CoordinateToPixel(i), CoordinateToPixel(0),
CoordinateToPixel(i), CoordinateToPixel(kBoardSize - 1));
line(CoordinateToPixel(0), CoordinateToPixel(i),
CoordinateToPixel(kBoardSize - 1), CoordinateToPixel(i));
}
// 绘制星位
int center = kBoardSize / 2;
int starPos[3][2] = {{center, center}, {3, 3}, {11, 3}, {3, 11}, {11, 11}};
for (int i = 0; i < 5; ++i) {
int x = starPos[i][0];
int y = starPos[i][1];
int px = CoordinateToPixel(x);
int py = CoordinateToPixel(y);
fillellipse(px, py, 5, 5);
}
}
// 绘制棋子
void DrawPiece(int x, int y, int color) {
int px = CoordinateToPixel(x);
int py = CoordinateToPixel(y);
setfillcolor(color == 1 ? BLACK : WHITE);
fillellipse(px, py, kRadius, kRadius);
}
int main() {
initgraph(kMargin * 2 + kGridSize * (kBoardSize - 1),
kMargin * 2 + kGridSize * (kBoardSize - 1));
settextstyle(20, 0, "Consolas");
DrawBoard(); // 绘制棋盘
int turn = 1; // 轮到黑方先手
while (true) {
// 读取鼠标点击事件
MOUSEMSG msg = GetMouseMsg();
if (msg.uMsg == WM_LBUTTONDOWN) {
int x = (msg.x - kMargin + kGridSize / 2) / kGridSize;
int y = (msg.y - kMargin + kGridSize / 2) / kGridSize;
if (x >= 0 && x < kBoardSize && y >= 0 && y < kBoardSize) {
if (board[x][y] == 0) {
// 没有落子,可以下棋
DrawPiece(x, y, turn == 1 ? 1 : 2);
board[x][y] = turn;
turn = 3 - turn; // 转换为对方下棋
} else {
// 该位置已经有棋子了,不能下棋
outtextxy(10, 10, "该位置已经有棋子了,请重新下棋!");
}
}
}
}
closegraph();
return 0;
}
```
这个代码只是一个简单的五子棋实现,还有很多细节需要完善,比如判断胜负、悔棋、重新开始等功能。
相关推荐
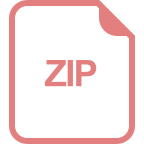













