如何利用编程将文件数据读入到Hbase表中
时间: 2024-02-23 09:02:14 浏览: 68
首先,你需要在编程语言中安装合适的 HBase 客户端库,比如 Java 语言中的 HBase API。接下来,你需要编写程序来读取文件数据,将其转换为 HBase 表中的行和列族,并将其插入到 HBase 表中。
下面是一个 Java 语言示例程序,可以将文件数据读入到 HBase 表中:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.Connection;
import org.apache.hadoop.hbase.client.ConnectionFactory;
import org.apache.hadoop.hbase.client.Put;
import org.apache.hadoop.hbase.client.Table;
import org.apache.hadoop.hbase.util.Bytes;
public class HBaseFileLoader {
public static void main(String[] args) throws IOException {
// 创建 HBase 配置对象
Configuration config = HBaseConfiguration.create();
// 创建 HBase 连接对象
Connection connection = ConnectionFactory.createConnection(config);
// 获取 HBase 表对象
TableName tableName = TableName.valueOf("table_name");
Table table = connection.getTable(tableName);
// 读取文件数据
BufferedReader reader = new BufferedReader(new FileReader("data.txt"));
String line;
while ((line = reader.readLine()) != null) {
// 将每行数据转换为 HBase 行
String[] fields = line.split(",");
String rowKey = fields[0];
Put put = new Put(Bytes.toBytes(rowKey));
put.addColumn(Bytes.toBytes("column_family"), Bytes.toBytes("column_name"), Bytes.toBytes(fields[1]));
// 将 HBase 行插入到表中
table.put(put);
}
// 关闭资源
reader.close();
table.close();
connection.close();
}
}
```
在上面的示例程序中,我们首先创建了 HBase 配置对象和连接对象,然后获取了需要插入数据的 HBase 表对象。接着,我们打开数据文件,循环读取每行数据,并将每行数据转换为 HBase 行。最后,我们将 HBase 行插入到表中,并关闭资源。
需要注意的是,在实际应用中,我们还需要对 HBase 表进行创建、删除、查询、修改等操作,以满足业务需求。
相关推荐
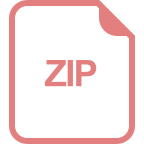
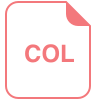
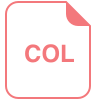
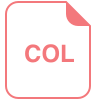
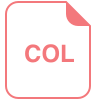
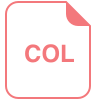









