以有序链表编制一个能演示执行集合的并、交和差运算的程序。
时间: 2024-04-29 09:26:03 浏览: 19
好的,我知道了。这是一个基本的数据结构问题,我可以为您提供一个示例程序来演示集合的并、交和差运算。以下是使用有序链表实现集合运算的示例程序:
```
#include <stdio.h>
#include <stdlib.h>
struct node
{
int data;
struct node *next;
};
void insert(struct node **head, int x)
{
struct node *ptr, *temp;
ptr = *head;
if (!ptr || ptr->data > x)
{
temp = (struct node *)malloc(sizeof(struct node));
temp->data = x;
temp->next = *head;
*head = temp;
}
else
{
while (ptr->next && ptr->next->data < x)
{
ptr = ptr->next;
}
temp = (struct node *)malloc(sizeof(struct node));
temp->data = x;
temp->next = ptr->next;
ptr->next = temp;
}
}
void display(struct node *head)
{
struct node *temp = head;
if (temp == NULL)
{
printf("List is empty\n");
}
else
{
while (temp != NULL)
{
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
}
struct node *set_union(struct node *a, struct node *b)
{
struct node *result = NULL;
struct node *tempA = a, *tempB = b;
while (tempA != NULL && tempB != NULL)
{
if (tempA->data == tempB->data)
{
insert(&result, tempA->data);
tempA = tempA->next;
tempB = tempB->next;
}
else if (tempA->data < tempB->data)
{
insert(&result, tempA->data);
tempA = tempA->next;
}
else
{
insert(&result, tempB->data);
tempB = tempB->next;
}
}
while (tempA != NULL)
{
insert(&result, tempA->data);
tempA = tempA->next;
}
while (tempB != NULL)
{
insert(&result, tempB->data);
tempB = tempB->next;
}
return result;
}
struct node *set_intersection(struct node *a, struct node *b)
{
struct node *result = NULL;
struct node *tempA = a, *tempB = b;
while (tempA != NULL && tempB != NULL)
{
if (tempA->data == tempB->data)
{
insert(&result, tempA->data);
tempA = tempA->next;
tempB = tempB->next;
}
else if (tempA->data < tempB->data)
{
tempA = tempA->next;
}
else
{
tempB = tempB->next;
}
}
return result;
}
struct node *set_difference(struct node *a, struct node *b)
{
struct node *result = NULL;
struct node *tempA = a, *tempB = b;
while (tempA != NULL && tempB != NULL)
{
if (tempA->data == tempB->data)
{
tempA = tempA->next;
tempB = tempB->next;
}
else if (tempA->data < tempB->data)
{
insert(&result, tempA->data);
tempA = tempA->next;
}
else
{
tempB = tempB->next;
}
}
while (tempA != NULL)
{
insert(&result, tempA->data);
tempA = tempA->next;
}
return result;
}
int main()
{
struct node *a = NULL, *b = NULL, *c = NULL;
insert(&a, 3);
insert(&a, 7);
insert(&a, 8);
insert(&a, 11);
insert(&b, 1);
insert(&b, 7);
insert(&b, 8);
insert(&b, 10);
printf("Set A: ");
display(a);
printf("Set B: ");
display(b);
c = set_union(a, b);
printf("Set A union B: ");
display(c);
c = set_intersection(a, b);
printf("Set A intersection B: ");
display(c);
c = set_difference(a, b);
printf("Set A difference B: ");
display(c);
return 0;
}
```
我希望这个程序可以帮到你。如果你有任何其他问题,请随时问我!
相关推荐
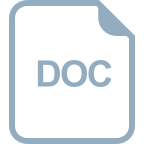














