self.setDragMode(QGraphicsView.ScrollHandDrag)
时间: 2024-02-02 07:49:48 浏览: 28
This line of code sets the drag mode of a QGraphicsView object to "ScrollHandDrag". This means that the user can drag the view around by clicking and dragging with the mouse button, as if they were scrolling the view by hand. This is a common feature in image viewing or map applications.
相关问题
将文件夹的图片生成到QtWidgets.QGraphicsView(self.centralwidget)
好的,我理解你的问题了。你想将一个文件夹中的所有图片显示在Qt的QGraphicsView中。我可以给你提供一些参考。
首先,你需要安装Pillow模块,这是Python的图像处理库。你可以使用pip命令来安装它:
```
pip install Pillow
```
然后,你需要编写代码来读取文件夹中的所有图片,创建QPixmap对象并将其添加到QGraphicsScene中。下面是一个简单的Python函数,它会读取一个文件夹中的所有图片,并将它们显示在QGraphicsView中:
```python
from PyQt5.QtWidgets import QGraphicsScene, QGraphicsPixmapItem
from PyQt5.QtGui import QPixmap
import os
def load_images(folder_path, scene):
# 遍历文件夹中的所有文件
for filename in os.listdir(folder_path):
# 如果是图片文件
if filename.endswith(".jpg") or filename.endswith(".png"):
# 创建QPixmap对象
pixmap = QPixmap(os.path.join(folder_path, filename))
# 创建QGraphicsPixmapItem对象
pixmap_item = QGraphicsPixmapItem(pixmap)
# 将QGraphicsPixmapItem对象添加到QGraphicsScene中
scene.addItem(pixmap_item)
```
在你的Qt应用程序中,你可以这样使用它:
```python
from PyQt5.QtWidgets import QMainWindow, QGraphicsView, QGraphicsScene
from PyQt5.QtCore import Qt
import sys
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建QGraphicsView和QGraphicsScene
self.view = QGraphicsView(self.centralwidget)
self.scene = QGraphicsScene(self.view)
self.view.setScene(self.scene)
# 加载图片
load_images("path/to/folder", self.scene)
# 设置QGraphicsView的属性
self.view.setRenderHint(QPainter.Antialiasing)
self.view.setDragMode(QGraphicsView.ScrollHandDrag)
self.view.setViewportUpdateMode(QGraphicsView.FullViewportUpdate)
self.view.setAlignment(Qt.AlignLeft | Qt.AlignTop)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
注意,这只是一个简单的示例,你可以根据你的需要进行修改和扩展。
qgraphicsview图像缩放
QGraphicsView 有一个 setTransform() 方法,可以用来设置 QGraphicsView 的缩放比例。下面是一个简单的示例代码,演示了如何使用 setTransform() 方法来缩放 QGraphicsView 中的图像:
```python
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QPixmap
from PyQt5.QtWidgets import QApplication, QGraphicsScene, QGraphicsView
class ImageViewer(QGraphicsView):
def __init__(self, parent=None):
super(ImageViewer, self).__init__(parent)
self.scene = QGraphicsScene(self)
self.setScene(self.scene)
self.image = QPixmap("path_to_image_file")
self.scene.addPixmap(self.image)
self.setDragMode(QGraphicsView.ScrollHandDrag)
self.setRenderHint(QPainter.Antialiasing)
self.setRenderHint(QPainter.SmoothPixmapTransform)
self.setTransformationAnchor(QGraphicsView.AnchorUnderMouse)
def wheelEvent(self, event):
# 缩放因子,这里设置为 1.2,可以根据需求调整
factor = 1.2
if event.angleDelta().y() > 0:
# 放大
self.scale(factor, factor)
else:
# 缩小
self.scale(1 / factor, 1 / factor)
if __name__ == '__main__':
app = QApplication([])
viewer = ImageViewer()
viewer.show()
app.exec_()
```
在上面的示例中,我们使用 QGraphicsScene 和 QGraphicsView 显示了一张图像。通过重写 QGraphicsView 的 wheelEvent() 方法,我们可以捕获鼠标滚轮事件,并根据滚动方向来放大或缩小图像。在缩放时,我们使用 setScale() 方法来设置缩放比例。通过设置 setTransformationAnchor() 方法,我们可以使缩放中心点位于鼠标指针下方。
相关推荐
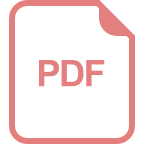
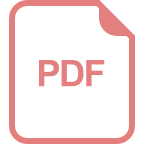












