每句代码带注解:1.构造学生信息链表,学生信息应包括姓名和年龄(链表初始化)char name(20) ;int age 2.根据自己的需要建立链表添加学生信息,学生信息不得少于3条(建立链表:头插法或者尾插法皆可) 3.打印出所有学生的信息(遍历),并统计学生人数(求链表长度) 4.在第二个位置插入一个学生信息,姓名为Tom,年龄为10(链表插入),并打印出插入后的学生信息表(遍历) 5.查找年龄为10的学生,打印其姓名(按值查找) 6.删除第二个学生的信息(删除),打印删除后的学生信息表(遍历)
时间: 2023-12-30 16:02:11 浏览: 27
1.构造学生信息链表,学生信息应包括姓名和年龄(链表初始化)char name(20) ;int age
```
//定义学生结构体
struct Student{
char name[20];
int age;
struct Student *next; //定义指向下一个学生的指针
};
//初始化链表
struct Student *head = NULL;
struct Student *tail = NULL;
```
2.根据自己的需要建立链表添加学生信息,学生信息不得少于3条(建立链表:头插法或者尾插法皆可)
```
//尾插法添加学生信息
struct Student *node1 = (struct Student*)malloc(sizeof(struct Student));
strcpy(node1->name, "Alice");
node1->age = 20;
node1->next = NULL; //尾节点的指针一定要赋为NULL
if(head == NULL){
head = node1;
tail = node1;
}
else{
tail->next = node1;
tail = node1;
}
struct Student *node2 = (struct Student*)malloc(sizeof(struct Student));
strcpy(node2->name, "Bob");
node2->age = 22;
node2->next = NULL;
tail->next = node2;
tail = node2;
struct Student *node3 = (struct Student*)malloc(sizeof(struct Student));
strcpy(node3->name, "Cathy");
node3->age = 21;
node3->next = NULL;
tail->next = node3;
tail = node3;
```
3.打印出所有学生的信息(遍历),并统计学生人数(求链表长度)
```
//遍历链表打印学生信息
int count = 0;
struct Student *p = head;
while(p != NULL){
printf("Name: %s, Age: %d\n", p->name, p->age);
count++;
p = p->next;
}
printf("Total number of students: %d\n", count);
```
4.在第二个位置插入一个学生信息,姓名为Tom,年龄为10(链表插入),并打印出插入后的学生信息表(遍历)
```
//在第二个位置插入学生信息
struct Student *node4 = (struct Student*)malloc(sizeof(struct Student));
strcpy(node4->name, "Tom");
node4->age = 10;
node4->next = NULL;
struct Student *p = head;
int i = 1;
while(i < 2 && p != NULL){ //找到要插入的位置
i++;
p = p->next;
}
node4->next = p->next;
p->next = node4;
//遍历链表打印学生信息
int count = 0;
p = head;
while(p != NULL){
printf("Name: %s, Age: %d\n", p->name, p->age);
count++;
p = p->next;
}
printf("Total number of students: %d\n", count);
```
5.查找年龄为10的学生,打印其姓名(按值查找)
```
//查找年龄为10的学生
struct Student *p = head;
while(p != NULL){
if(p->age == 10){
printf("Name: %s\n", p->name);
break;
}
p = p->next;
}
```
6.删除第二个学生的信息(删除),打印删除后的学生信息表(遍历)
```
//删除第二个学生信息
struct Student *p = head;
int i = 1;
while(i < 2 && p != NULL){ //找到要删除的位置
i++;
p = p->next;
}
struct Student *q = p->next;
p->next = q->next;
free(q);
//遍历链表打印学生信息
int count = 0;
p = head;
while(p != NULL){
printf("Name: %s, Age: %d\n", p->name, p->age);
count++;
p = p->next;
}
printf("Total number of students: %d\n", count);
```
相关推荐
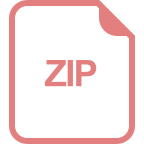
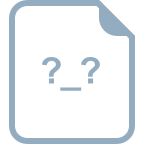
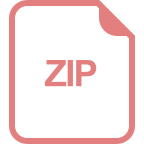














