c++中,有一个类,包含多个double类型的成员变量;现在有一个类数组,要对这个类数组进行遍历,把每个类的成员变量保存到对应的数组中,且每个数组都对应好成员变量名
时间: 2023-08-16 21:09:22 浏览: 87
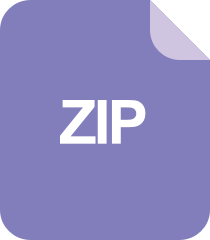
C++ 包含类两个类互相调用彼此的类成员变量和方法
您可以使用模板元编程和反射来实现这个功能。下面是一个简单的例子:
```c++
#include <iostream>
#include <string>
#include <array>
template <class T>
struct Reflection {
static constexpr size_t size = sizeof(T) / sizeof(double);
static std::array<double, size> values;
static std::array<std::string, size> names;
};
template <class T>
std::array<double, Reflection<T>::size> Reflection<T>::values{};
template <class T>
std::array<std::string, Reflection<T>::size> Reflection<T>::names{};
struct MyClass {
double a = 1.0;
double b = 2.0;
double c = 3.0;
};
template <>
struct Reflection<MyClass> {
static constexpr size_t size = sizeof(MyClass) / sizeof(double);
static std::array<double, size> values;
static std::array<std::string, size> names;
};
template <>
std::array<double, Reflection<MyClass>::size> Reflection<MyClass>::values{};
template <>
std::array<std::string, Reflection<MyClass>::size> Reflection<MyClass>::names{ "a", "b", "c" };
template <class T, size_t N>
void traverse(const std::array<T, N>& arr, std::array<std::array<double, Reflection<T>::size>, N>& values, std::array<std::array<std::string, Reflection<T>::size>, N>& names) {
for (size_t i = 0; i < N; ++i) {
const T& obj = arr[i];
for (size_t j = 0; j < Reflection<T>::size; ++j) {
values[i][j] = obj.*reinterpret_cast<const double*>(&Reflection<T>::values[j]);
names[i][j] = Reflection<T>::names[j];
}
}
}
int main() {
std::array<MyClass, 3> arr{ MyClass{1.0, 2.0, 3.0}, MyClass{4.0, 5.0, 6.0}, MyClass{7.0, 8.0, 9.0} };
std::array<std::array<double, Reflection<MyClass>::size>, 3> values{};
std::array<std::array<std::string, Reflection<MyClass>::size>, 3> names{};
traverse(arr, values, names);
for (size_t i = 0; i < 3; ++i) {
std::cout << "Object " << i << ":" << std::endl;
for (size_t j = 0; j < Reflection<MyClass>::size; ++j) {
std::cout << " " << names[i][j] << ": " << values[i][j] << std::endl;
}
}
return 0;
}
```
在这个例子中,我们定义了一个名为 `MyClass` 的类,它有三个成员变量。我们还定义了一个名为 `Reflection` 的类模板,它有两个静态成员变量 `values` 和 `names`,它们分别保存了类的成员变量的值和名称。我们使用了一个特化版本的 `Reflection` 模板来处理 `MyClass` 类型。
在 `main` 函数中,我们创建了一个 `MyClass` 类型的对象数组 `arr`,并使用 `traverse` 函数来将每个对象的成员变量的值保存到对应的数组中,同时将成员变量名保存到对应的数组中。最后,我们遍历保存成员变量值的数组和保存成员变量名的数组,并输出它们的值。
这个例子只是一个简单的实现,您可以根据自己的需求来修改代码。
阅读全文
相关推荐
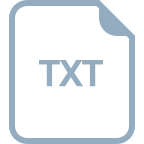
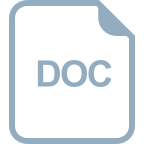



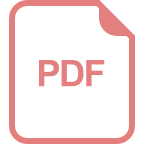
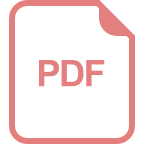
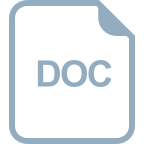
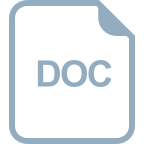
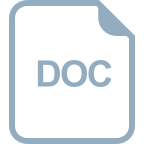
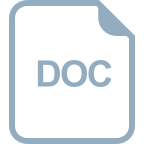
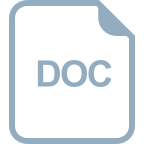
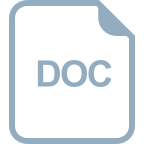
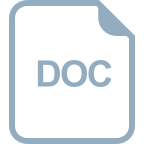
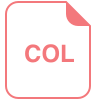

