1、 用c语言程序实现波兰符号法。 实现功能:输入波兰符号法公式,求出公式类型 输入:波兰符号法的命题公式 输出:输出公式类型。
时间: 2024-02-23 09:00:05 浏览: 90
以下是一个简单的C语言程序,实现了波兰符号法的计算和公式类型判断:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <string.h>
#define MAX_EXPR_LEN 100
typedef struct _stack {
int top;
int data[MAX_EXPR_LEN];
} Stack;
void init_stack(Stack *stack) {
stack->top = -1;
}
int is_empty(Stack *stack) {
return stack->top == -1;
}
int is_full(Stack *stack) {
return stack->top == MAX_EXPR_LEN - 1;
}
void push(Stack *stack, int value) {
if (is_full(stack)) {
printf("Stack is full!\n");
exit(EXIT_FAILURE);
}
stack->top++;
stack->data[stack->top] = value;
}
int pop(Stack *stack) {
if (is_empty(stack)) {
printf("Stack is empty!\n");
exit(EXIT_FAILURE);
}
int value = stack->data[stack->top];
stack->top--;
return value;
}
int evaluate(char *expr) {
Stack stack;
init_stack(&stack);
int i, op1, op2;
char ch;
for (i = 0; expr[i] != '\0'; i++) {
ch = expr[i];
if (isdigit(ch)) {
push(&stack, ch - '0');
} else {
op2 = pop(&stack);
op1 = pop(&stack);
switch (ch) {
case '+':
push(&stack, op1 + op2);
break;
case '-':
push(&stack, op1 - op2);
break;
case '*':
push(&stack, op1 * op2);
break;
case '/':
push(&stack, op1 / op2);
break;
default:
printf("Invalid operator!\n");
exit(EXIT_FAILURE);
}
}
}
return pop(&stack);
}
int get_expr_type(char *expr) {
if (strlen(expr) == 1 && !isdigit(expr[0])) {
return -1; // 单个符号
}
int i, num_count = 0, op_count = 0;
char ch;
for (i = 0; expr[i] != '\0'; i++) {
ch = expr[i];
if (isdigit(ch)) {
num_count++;
} else {
op_count++;
}
}
if (num_count == op_count + 1) {
return 0; // 合法的波兰符号法表达式
} else {
return 1; // 非法表达式
}
}
int main() {
char expr[MAX_EXPR_LEN];
printf("Enter a Polish notation expression: ");
scanf("%s", expr);
int type = get_expr_type(expr);
if (type == 0) {
int result = evaluate(expr);
printf("Result: %d\n", result);
} else {
printf("Invalid expression!\n");
}
return 0;
}
```
这个程序首先定义了一个栈结构体,实现了栈的基本操作,包括初始化、判断栈是否为空或已满、入栈、出栈等。然后定义了两个函数,`evaluate`函数用于计算波兰符号法表达式的值,`get_expr_type`函数用于判断表达式类型(单个符号、合法的波兰符号法表达式或非法表达式)。
在`main`函数中,首先读入一个波兰符号法表达式,并调用`get_expr_type`函数判断其类型。如果是合法的波兰符号法表达式,则调用`evaluate`函数计算其值并输出结果;否则输出错误信息。
相关推荐
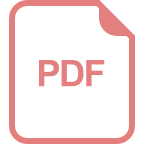
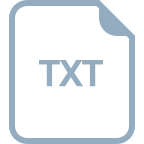












