import os import pydicom from PIL import Image # 将某个 IMA 文件转换为 PNG 格式并保存 def save_as_png(ds, png_path): # 将 IMA 像素数据转换为图像 pixel_data = ds.pixel_array image = Image.fromarray(pixel_data) image = image.convert('L') # 灰度化 # 保存为 PNG 格式 image.save(png_path) # 定义输入和输出目录 input_dir = './data' output_dir = './output' # 遍历每个子目录 for subdir in os.listdir(input_dir): subdir_path = os.path.join(input_dir, subdir) if not os.path.isdir(subdir_path): continue print(f'Processing {subdir}') # 在输出目录中创建相同的子目录 out_subdir_path = os.path.join(output_dir, subdir) os.makedirs(out_subdir_path, exist_ok=True) # 遍历 full_3mm 和 quarter_3mm 文件夹 for mode in ['full_3mm', 'quarter_3mm']: mode_path = os.path.join(subdir_path, mode) if not os.path.isdir(mode_path): continue # 遍历每个 IMA 文件并转换为 PNG 格式 for filename in os.listdir(mode_path): if not filename.endswith('.ima'): continue ima_path = os.path.join(mode_path, filename) ds = pydicom.dcmread(ima_path) # png_filename = f'{subdir}_{mode}_{ds.SOPInstanceUID}.png' # 使用所有信息作为文件名 png_filename = os.path.splitext(filename)[0] + '.png' png_path = os.path.join(out_subdir_path, png_filename) save_as_png(ds, png_path)为什么无生成
时间: 2024-04-02 10:34:12 浏览: 103
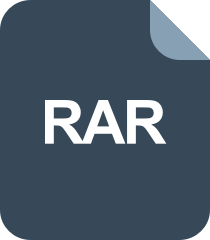
PNG.rar_PGN_png_png读取_读 png_读取png
这段代码可能无法生成PNG文件是因为文件保存路径不存在或没有写入权限。你需要检查输出目录是否存在,如果不存在则需要使用`os.makedirs()`创建目录。同时,你也需要检查代码中使用的输出文件名是否正确,如果文件名中包含非法字符或长度过长,可能会导致文件无法保存。你可以添加一些调试代码,例如调用`os.path.exists()`检查文件路径是否存在,以及添加异常处理代码,以便在保存失败时输出错误信息。
阅读全文
相关推荐
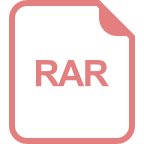
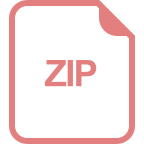
















