写一段基于linux的can通信代码
时间: 2024-04-08 09:29:43 浏览: 66
当涉及到基于Linux的CAN通信代码时,可以使用SocketCAN接口来实现。下面是一个简单的示例代码,用于发送和接收CAN消息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <net/if.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <sys/ioctl.h>
#include <linux/can.h>
#include <linux/can/raw.h>
int main() {
int s; // 套接字描述符
struct sockaddr_can addr;
struct ifreq ifr;
struct can_frame frame;
int nbytes;
// 创建CAN套接字
s = socket(PF_CAN, SOCK_RAW, CAN_RAW);
if (s == -1) {
perror("Error while opening socket");
return -1;
}
// 设置CAN接口名
strcpy(ifr.ifr_name, "can0");
ioctl(s, SIOCGIFINDEX, &ifr);
// 绑定CAN套接字到接口
addr.can_family = AF_CAN;
addr.can_ifindex = ifr.ifr_ifindex;
if (bind(s, (struct sockaddr *)&addr, sizeof(addr)) == -1) {
perror("Error in socket bind");
return -2;
}
// 准备CAN帧
frame.can_id = 0x123; // CAN标识符
frame.can_dlc = 8; // 数据长度
sprintf(frame.data, "Hello!");
// 发送CAN帧
nbytes = write(s, &frame, sizeof(struct can_frame));
if (nbytes == -1) {
perror("Error in socket write");
return -3;
}
printf("Sent CAN frame with ID: 0x%03X\n", frame.can_id);
// 接收CAN帧
nbytes = read(s, &frame, sizeof(struct can_frame));
if (nbytes == -1) {
perror("Error in socket read");
return -4;
}
printf("Received CAN frame with ID: 0x%03X, Data: %s\n", frame.can_id, frame.data);
// 关闭套接字
close(s);
return 0;
}
```
请注意,上述代码假设已经在系统上配置了CAN接口(例如can0)。确保在编译时链接`-lsocketcan`库。
这是一个简单的示例,用于发送一帧CAN消息并接收一帧CAN消息。你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
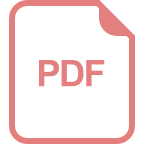
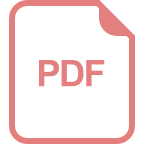
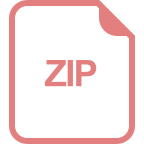
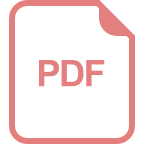
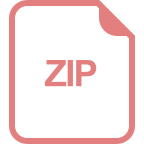
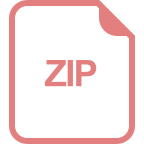
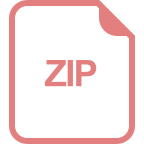
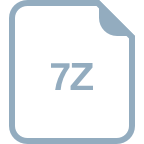
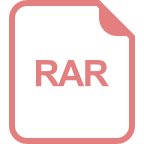
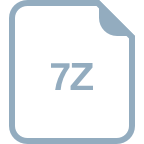
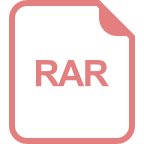
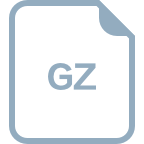
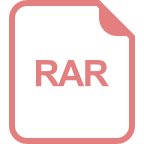
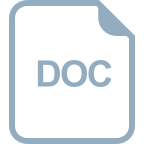
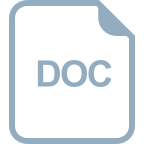
