编一段CAN通信的代码
时间: 2023-10-24 09:08:49 浏览: 84
当然可以!以下是一个简单的CAN通信的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <net/if.h>
#include <sys/ioctl.h>
#include <sys/socket.h>
#include <linux/can.h>
#include <linux/can/raw.h>
int main() {
int s;
struct sockaddr_can addr;
struct ifreq ifr;
struct can_frame frame;
// 创建Socket
s = socket(PF_CAN, SOCK_RAW, CAN_RAW);
if (s < 0) {
perror("Error while opening socket");
return -1;
}
// 设置接口名称
strcpy(ifr.ifr_name, "can0");
ioctl(s, SIOCGIFINDEX, &ifr);
// 绑定Socket到CAN接口
addr.can_family = AF_CAN;
addr.can_ifindex = ifr.ifr_ifindex;
if (bind(s, (struct sockaddr *)&addr, sizeof(addr)) < 0) {
perror("Error in socket bind");
return -2;
}
// 准备CAN帧
frame.can_id = 0x123; // CAN帧的ID
frame.can_dlc = 8; // 数据长度为8字节
sprintf(frame.data, "HelloCAN");
// 发送CAN帧
if (write(s, &frame, sizeof(struct can_frame)) != sizeof(struct can_frame)) {
perror("Error in socket write");
return -3;
}
printf("CAN frame sent\n");
close(s);
return 0;
}
```
这段代码使用了Linux下的Socket编程接口,通过CAN套接字进行CAN通信。其中,需要根据实际情况修改接口名称("can0")和CAN帧的ID(0x123)等参数。
请注意,这只是一个简单的示例,实际的CAN通信可能涉及更多的配置和处理逻辑。为了能够成功运行此代码,您需要在Linux系统上安装并配置CAN接口,并确保具备相应的权限。
希望这段代码能够帮助到您!如果您有更多问题,请随时提问。
阅读全文
相关推荐
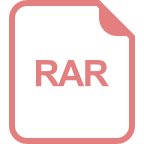









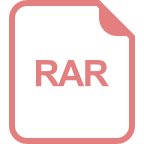
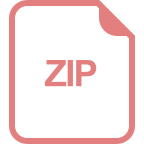
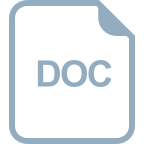


