解释一下 public void testUnKownCode(){ List<MachineRecordModel> list = machineRecordService.getListByUnkownGoodsCode(); List<String> goodsCodes = list.stream().map(MachineRecordModel::getCodeContent).collect(Collectors.toList()); List<TextDataModel> existsList = tempTextDataService.getListByGoodsCodes(goodsCodes); Set<String> existsCodesSet = existsList.stream().map(TextDataModel::getGoodBar).collect(Collectors.toSet()); List<TextDataModel> needInsertList = goodsCodes.stream().filter(code -> !existsCodesSet.contains(code)) .map(code -> { TextDataModel textDataModel = new TextDataModel(); textDataModel.setGoodBar(code); textDataModel.setHas(0); return textDataModel; }).collect(Collectors.toList()); tempTextDataService.saveBatch(needInsertList); System.out.println(""); }
时间: 2023-05-22 15:04:59 浏览: 92
这段代码是一个 Java 中的方法,这个方法名是 testUnKownCode,它的访问修饰符是 public,返回值类型是 void,也就是不返回任何值。这个方法会调用 machineRecordService 的 getListByUnkownGoodsCode 方法,返回一个 MachineRecordModel 类型的列表。然后利用 Java 8 的 Stream 特性,将 MachineRecordModel 类型的列表转换为一个 String 类型的列表。接着,使用得到的 String 类型列表,调用 tempTextDataService 的 getListByGoodsCodes 方法,返回一个 TextDataModel 类型的列表。再次使用 Stream 特性,将这个 TextDataModel 类型的列表转换为一个 String 类型的 HashSet(无序集合)。利用 Stream 特性,过滤出需要插入的数据,并且将字符串类型的数据转换为一个 TextDataModel 类型的列表,然后调用 tempTextDataService 的 saveBatch 方法批量保存这些数据。最后,这个方法会输出一个空字符串。
相关问题
List<MessageDO> results0 = Collections.emptyList();List<MessageDO> results1 = Collections.emptyList();List<MessageDO> results2 = Collections.emptyList();把result0,result1和result2合并
您可以使用Java 8的Stream API中的Stream.concat()方法来合并多个List。具体的代码实现可以参考下面的示例:
```java
import java.util.Collections;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class MergeListsExample {
public static void main(String[] args) {
// 假设有三个List
List<MessageDO> results0 = Collections.emptyList();
List<MessageDO> results1 = Collections.emptyList();
List<MessageDO> results2 = Collections.emptyList();
// 合并多个List
List<MessageDO> mergedList = Stream.concat(
Stream.concat(results0.stream(), results1.stream()), results2.stream())
.collect(Collectors.toList());
// 输出合并后的List
System.out.println(mergedList);
}
}
```
注意,上述示例中的results0、results1和results2是您需要合并的三个List。您可以将它们替换为您的三个List,然后使用Stream.concat()方法将它们合并到一个Stream中。合并后的Stream可以使用collect方法和Collectors.toList()收集器进行收集。
/**循环递归**/ public List<PrpdCompanyVo> deptList(String comCode) { /**查询指定公司代码的**/ List<PrpdCompanyPo> list0 = this.selectByPid(comCode); PrpdCompanyVo prpdCompanyVo = new PrpdCompanyVo(); prpdCompanyVo.setComCode(comCode); prpdCompanyVo.setIsEnable("Y"); if(CollectionUtils.isEmpty(list0)){ List<PrpdCompanyVo> listVo = new ArrayList<>(); listVo.add(prpdCompanyVo); return listVo; //根节点是空 //返回自己 } List<PrpdCompanyVo> voList=list0.stream().map(this::converVO).collect(Collectors.toList()); List<PrpdCompanyVo> voListBackup =list0.stream().map(this::converVO).collect(Collectors.toList()); voListBackup.forEach(item-> buildChild(item,voList)); return voList; } private void buildChild(PrpdCompanyVo item, List<PrpdCompanyVo> voListO){ List<PrpdCompanyPo> listChild = this.selectByPid(item.getComCode()); if(CollectionUtils.isEmpty(listChild)){ voListO.add(item); return; } List<PrpdCompanyVo> voList=listChild.stream().map(this::converVO).collect(Collectors.toList()); item.setChildList(voList); voList.forEach(item2-> buildChild(item2,voListO)); } private PrpdCompanyVo converVO(PrpdCompanyPo po){ PrpdCompanyVo VO = new PrpdCompanyVo(); BeanUtils.copyProperties(po,VO); return VO; }
这段代码是一个 Java 方法的示例,用于实现循环递归查询部门列表。代码中使用了一个 `PrpdCompanyPo` 类表示公司信息,使用了一个 `PrpdCompanyVo` 类表示返回的部门列表。
方法名为 `deptList`,接受一个参数 `comCode`,表示公司代码。该方法首先通过调用 `selectByPid` 方法查询指定公司代码的下级公司列表,并将其转换为 `PrpdCompanyVo` 对象的列表。
如果下级公司列表为空,说明当前公司是根节点,将其添加到结果列表中并返回。
如果下级公司列表不为空,将其转换为 `PrpdCompanyVo` 对象的列表,并遍历每个下级公司。对每个下级公司,再次调用 `selectByPid` 方法查询其下级公司列表,并将其转换为 `PrpdCompanyVo` 对象的列表。将这个列表设置为当前公司的子部门列表,并递归调用 `buildChild` 方法处理该子部门列表。
`buildChild` 方法也是一个递归方法,用于构建子部门列表。如果子部门列表为空,将当前部门添加到结果列表中并返回。如果子部门列表不为空,将其转换为 `PrpdCompanyVo` 对象的列表,并对每个子部门递归调用 `buildChild` 方法处理。
最后,通过调用 `converVO` 方法将 `PrpdCompanyPo` 对象转换为 `PrpdCompanyVo` 对象。
请注意,这只是一个示例代码,具体的实现可能需要根据您的数据结构和业务需求进行适当地修改。希望这对您有所帮助!如果您有其他问题,请随时提问。
阅读全文
相关推荐
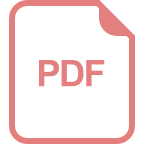
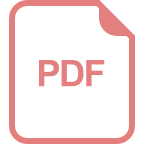
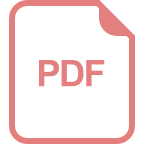













