按照C/S模型编写两个程序,一个是服务器程序,一个是客户端程序。设计的程序包含以下功能: 1.Windows环境下运行,并有显示界面。 2.服务器端监听端口,有客户请求时就显示客户端IP地址、端口和收到的数据。
时间: 2024-02-06 18:09:46 浏览: 53
好的,我可以为您编写这样的程序。因为您没有指定编程语言,我将使用Python语言来实现。
首先,我们需要安装Python的图形用户界面工具包Tkinter。在Windows下,打开命令提示符,输入以下命令来安装:
```
pip install tkinter
```
接下来,我们编写服务器端程序。服务器端程序需要监听一个端口,并在有客户请求时显示客户端IP地址、端口和收到的数据。
``` python
import socket
import threading
import tkinter as tk
class Server:
def __init__(self, host, port):
self.host = host
self.port = port
self.clients = []
self.running = False
self.window = tk.Tk()
self.window.title('服务器')
self.listbox = tk.Listbox(self.window, width=50)
self.listbox.pack(padx=10, pady=10)
self.start_button = tk.Button(self.window, text='启动', command=self.start)
self.start_button.pack(pady=10)
self.stop_button = tk.Button(self.window, text='停止', command=self.stop, state='disabled')
self.stop_button.pack(pady=10)
self.window.mainloop()
def start(self):
self.running = True
self.start_button.config(state='disabled')
self.stop_button.config(state='normal')
self.socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
self.socket.bind((self.host, self.port))
self.socket.listen()
self.thread = threading.Thread(target=self.listen_for_clients)
self.thread.start()
def stop(self):
self.running = False
self.socket.close()
def listen_for_clients(self):
while self.running:
client_socket, client_address = self.socket.accept()
self.clients.append(client_socket)
threading.Thread(target=self.handle_client, args=(client_socket, client_address)).start()
def handle_client(self, client_socket, client_address):
while self.running:
try:
data = client_socket.recv(1024).decode('utf-8')
if data:
self.listbox.insert(tk.END, f'{client_address[0]}:{client_address[1]}: {data}')
except:
break
self.clients.remove(client_socket)
client_socket.close()
server = Server('127.0.0.1', 8000)
```
在代码中,我们定义了一个Server类,该类包含以下方法:
- `__init__(self, host, port)`:构造方法,初始化主机和端口,并创建GUI界面。
- `start(self)`:启动服务器,开始监听端口。
- `stop(self)`:停止服务器,关闭socket连接。
- `listen_for_clients(self)`:监听客户端连接并创建线程处理客户端请求。
- `handle_client(self, client_socket, client_address)`:处理客户端请求。
在`__init__`方法中,我们创建了一个Tkinter窗口,并添加了两个按钮(启动和停止按钮)和一个列表框用于显示客户端IP地址、端口和收到的数据。
在`start`方法中,我们创建了一个socket对象,并调用`listen`方法开始监听端口。然后启动一个新线程来监听客户端连接。
在`listen_for_clients`方法中,我们使用一个while循环来监听客户端连接,当有新的客户端连接时,我们将客户端socket对象添加到`self.clients`列表中,并启动一个新线程来处理该客户端的请求。
在`handle_client`方法中,我们使用一个while循环来监听客户端发送的数据,当有数据到达时,我们将数据显示在列表框中。如果客户端断开连接或发生异常,我们将客户端socket对象从列表中移除,并关闭socket连接。
接下来,我们编写客户端程序。客户端程序需要连接到服务器端,并发送数据。我们可以使用Python的socket模块来实现。
``` python
import socket
import tkinter as tk
class Client:
def __init__(self, host, port):
self.host = host
self.port = port
self.window = tk.Tk()
self.window.title('客户端')
self.input_text = tk.Entry(self.window, width=50)
self.input_text.pack(padx=10, pady=10)
self.send_button = tk.Button(self.window, text='发送', command=self.send_message)
self.send_button.pack(pady=10)
self.window.mainloop()
def send_message(self):
message = self.input_text.get()
self.input_text.delete(0, tk.END)
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
s.connect((self.host, self.port))
s.sendall(message.encode('utf-8'))
client = Client('127.0.0.1', 8000)
```
在代码中,我们定义了一个Client类,该类包含以下方法:
- `__init__(self, host, port)`:构造方法,初始化主机和端口,并创建GUI界面。
- `send_message(self)`:发送消息到服务器端。
在`__init__`方法中,我们创建了一个Tkinter窗口,并添加了一个文本框和一个发送按钮。当用户点击发送按钮时,我们将文本框中的内容发送到服务器端。
在`send_message`方法中,我们创建了一个socket对象,并调用`connect`方法连接到服务器端。然后将消息发送到服务器端。
现在,我们可以在两台计算机上分别运行服务器端和客户端程序,并进行通信。当客户端发送数据时,服务器端将显示客户端IP地址、端口和收到的数据。
阅读全文
相关推荐
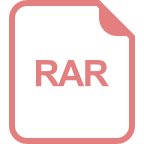
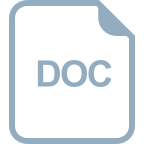
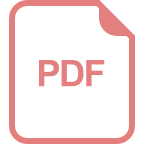
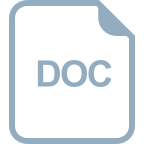
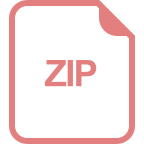
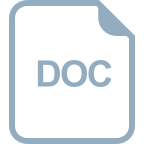
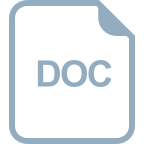
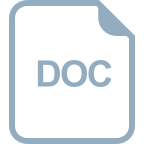
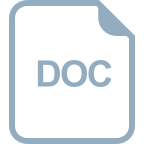
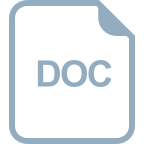
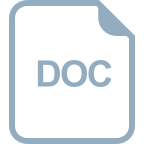


