print(f'句子:"{sentence}"的概率为: {probability:.3g}')什么作用
时间: 2023-12-06 14:42:55 浏览: 24
这行代码的作用是将一个字符串(变量名为 `sentence`)和一个浮点数(变量名为 `probability`)格式化输出到控制台上。其中,字符串被插入到大括号 `{}` 中,而浮点数则使用了格式化字符串中的 `.3g`,表示输出三位有效数字的科学计数法(如果数值小于1,则用小数的形式输出;如果数值大于等于1,则用科学计数法输出)。这个代码的作用是用来输出一个模型对于输入句子的预测概率。
相关问题
TypeError: Tokenizer.cut() missing 1 required positional argument: sentence
TypeError: Tokenizer.cut() missing 1 required positional argument: 'sentence'是因为在调用Tokenizer.cut()方法时没有传入必需的参数'sentence'导致的错误。要解决这个问题,你需要确保在调用cut()方法时传入正确的参数。
以下是一个示例,演示了如何使用Tokenizer.cut()方法并传入正确的参数'sentence':
```python
from jieba import Tokenizer
tokenizer = Tokenizer()
sentence = "我爱自然语言处理"
result = tokenizer.cut(sentence)
print(list(result))
```
在这个示例中,我们首先导入了Tokenizer类,并创建了一个Tokenizer对象。然后,我们定义了一个字符串变量sentence,它包含了要进行分词的句子。接下来,我们调用了Tokenizer对象的cut()方法,并将句子作为参数传入。最后,我们将分词结果转换为列表并打印出来。
请注意,为了正确使用Tokenizer.cut()方法,你需要先安装jieba库。你可以使用以下命令来安装jieba库:
```shell
pip install jieba
```
sentence-bert: sentence embeddings using siamese bert-networks emnlp
Sentence-BERT(双塔BERT网络)是一种使用BERT网络生成句子嵌入的方法。 该技术在自然语言处理领域中具有广泛的应用,并且与传统的词向量相比,它提供了更丰富和有趣的语义信息。 在这个技术中,双塔BERT网络被用来生成两个句子之间的相似性分数,这对于许多任务都非常有用。常见的应用包括文本相似度、信息检索和自然语言推理等。接着,我们需要将这个分数转换成一个向量,通过这个向量可以减少噪音,强化特征的相似性. 同时,这个向量可以被用来评估两个句子之间的相似性。 Sentence-BERT可以用于在许多领域进行嵌入学习,例如推荐系统、机器翻译、信息抽取和事实验证等。 此外,这种方法可在文本的特定领域中预先训练句子嵌入,从而在特定任务上提供更好的表现。 总之,Sentence-BERT是一种非常有用的技术,可以从不同角度对文本进行分析,并提供了许多在信息检索、自然语言理解和情感分析等领域中有用的功能。
相关推荐
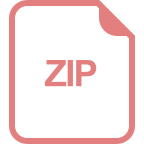
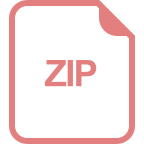












