DPC python
时间: 2023-10-15 15:26:40 浏览: 46
DPC(Dynamic Programming in Python)是一种使用动态规划算法解决问题的方法。它通过将复杂问题拆分为更小的子问题,并存储子问题的解决方案,从而避免重复计算,提高效率。在Python中,我们可以使用递归或迭代的方式来实现动态规划算法。你可以提供更具体的问题或情景,我将为你提供相应的示例代码和解释。
相关问题
dpc算法python
DPC算法是一种基于密度峰值聚类的聚类算法,可以有效地解决一些传统聚类算法无法处理的问题。在Python中,可以使用scikit-learn库中实现的DBSCAN算法来实现DPC算法。
首先,需要导入sklearn.cluster模块中的DBSCAN类。然后,可以使用DBSCAN类的fit方法对数据进行聚类。在聚类过程中,需要设置eps和min_samples两个参数,分别代表领域半径和最小样本数。可以通过调整这两个参数来获得更好的聚类效果。聚类结果可以通过DBSCAN类的labels_属性获取。
在使用DPC算法时,需要注意数据的预处理和参数的调整,以获得最佳的聚类效果。另外,由于DPC算法对噪声敏感,需要对噪声进行特殊处理,可以将噪声归为一个簇或者剔除噪声数据。综上所述,DPC算法是一种实用的聚类算法,可以在Python中方便地实现。
DPC算法 python
DPC(Density Peak Clustering)算法是一种基于密度的聚类算法,可以有效地处理具有不同密度的数据集。下面是一个简单的 Python 实现:
```python
import numpy as np
def dpc(data, dc, rho):
# 计算样本之间的距离矩阵
dist = np.sqrt(np.sum((data[:, np.newaxis, :] - data[np.newaxis, :, :]) ** 2, axis=2))
# 计算每个样本的局部密度
rho = np.sum(dist < dc, axis=1)
# 找到密度更高的样本作为核心点
core_points = np.where(rho > np.mean(rho))[0]
# 计算每个核心点到其它点的距离
core_dist = np.min(dist[core_points][:, core_points], axis=1)
# 找到每个样本的最近的核心点
nearest_core_points = core_points[np.argmin(dist[core_points][:, core_points], axis=1)]
# 找到每个样本的密度更高的近邻
higher_density_neighbors = np.where(rho > rho[nearest_core_points])[0]
# 找到每个样本的密度更高的近邻中距离最远的点
max_dist_neighbors = higher_density_neighbors[np.argmax(dist[higher_density_neighbors][:, nearest_core_points], axis=1)]
# 划分簇
clusters = {}
for i, p in enumerate(data):
if i not in core_points:
# 不是核心点的样本归类到最近的核心点所在的簇
c = nearest_core_points[i]
if c not in clusters:
clusters[c] = []
clusters[c].append(i)
else:
# 核心点归类到距离最近的核心点所在的簇
c = core_points[np.argmin(core_dist[core_points])]
if c not in clusters:
clusters[c] = []
clusters[c].append(i)
return clusters
```
其中,`data` 是一个 $n \times m$ 的数组,表示 $n$ 个 $m$ 维的数据点;`dc` 是一个阈值,用于确定核心点;`rho` 是一个 $n$ 维的数组,表示每个样本的局部密度。函数返回一个字典,表示划分的簇。
相关推荐
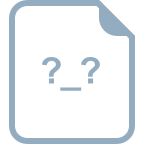
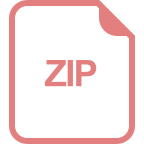
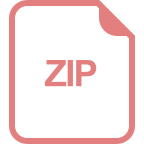












