用c语言写一个串口的代码
时间: 2024-02-15 16:05:32 浏览: 25
好的,下面是一个简单的串口通信的 C 语言代码,可以发送和接收数据:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
#include <string.h>
int main()
{
int fd; // 文件描述符
char buffer[256];
struct termios tty;
fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1)
{
perror("open_port: Unable to open /dev/ttyUSB0 - ");
return 1;
}
memset(&tty, 0, sizeof(tty));
if (tcgetattr(fd, &tty) != 0)
{
perror("tcgetattr error");
return 1;
}
cfsetospeed(&tty, B9600); // 设置波特率为 9600
cfsetispeed(&tty, B9600);
tty.c_cflag &= ~PARENB; // 无奇偶校验
tty.c_cflag &= ~CSTOPB; // 1 位停止位
tty.c_cflag |= CS8; // 8 位数据位
tty.c_cflag &= ~CRTSCTS; // 关闭硬件流控制
tty.c_lflag = 0; // 不使用终端模式
tty.c_cc[VMIN] = 1; // 非规范模式读取时的最小字符数
tty.c_cc[VTIME] = 0; // 非规范模式读取时的等待时间
if (tcsetattr(fd, TCSANOW, &tty) != 0)
{
perror("tcsetattr error");
return 1;
}
while (1)
{
printf("Enter your message: ");
fgets(buffer, sizeof(buffer), stdin);
int n = write(fd, buffer, strlen(buffer));
if (n < 0)
{
perror("write error");
return 1;
}
memset(buffer, 0, sizeof(buffer));
n = read(fd, buffer, sizeof(buffer));
if (n < 0)
{
perror("read error");
return 1;
}
printf("Received message: %s\n", buffer);
}
close(fd);
return 0;
}
```
该代码使用 Linux 的串口设备文件 /dev/ttyUSB0 进行通信,如果你使用的是 Windows 系统,需要使用相应的串口设备名称。另外,你需要根据实际情况设置波特率、数据位、停止位等参数。
相关推荐
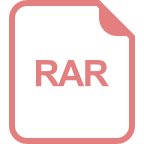














