a `vagrantfile` has been placed in this directory. you are now ready to `vagrant up` your first virtual environment! please read the comments in the vagrantfile as well as documentation on `vagrantup.com` for more information on using vagrant.
时间: 2023-05-01 19:05:54 浏览: 96
在这个目录下已经放置了一个叫做 `vagrantfile` 的文件。你现在可以准备启动你的第一个虚拟环境了!请阅读 `vagrantfile` 中的注释,以及在 `vagrantup.com` 上的文档,获取更多有关使用 vagrant 的信息。
相关问题
Consider defining a bean of type 'com.lsx.feign.client.UserClient' in your configuration.
This error message usually appears when you are using Spring Framework and you have not defined a bean of type `com.lsx.feign.client.UserClient` in your configuration.
To resolve this issue, you need to define a bean of type `UserClient` in your configuration. There are different ways to achieve this:
1. Use the `@Component` annotation on the `UserClient` interface or implementation class. This will automatically create a bean of this type.
2. Use the `@Bean` annotation to define a method that creates an instance of `UserClient` and returns it. This method can be placed in a configuration class annotated with `@Configuration`.
3. Use the `@Autowired` annotation to inject an instance of `UserClient` into your class. This assumes that there is a bean of type `UserClient` available in your application context.
Once you have defined a bean of type `UserClient`, the error message should disappear and your application should work as expected.
Your top-level group Ali-partners is over the 5 user limit and has been placed in a read-only state. To remove the read-only state and regain write access, ask your top-level group owner(s) to reduce the number of users in your top-level group to 5 users or less, or to upgrade to a paid tier which do not have user limits.
这是GitLab的限制规则之一,即免费版的顶级组织(top-level group)最多只能添加5个用户,超过限制则会将该组织设置为只读状态。要解除只读状态并恢复写访问权限,需请组织拥有者减少组织中用户的数量至5个或以下,或者升级到不受用户限制的付费版。付费版提供了更多功能和更高级别的支持,可以根据具体需求选择适合的版本。
相关推荐
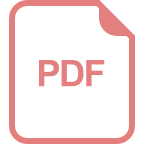
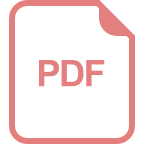
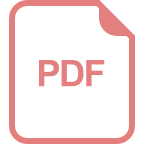













