Please write a simply code in python, the requirement is as followed: The Tower of Hanoi is a mathematical game or puzzle. It consists of three rods, and a number of disks of different sizes which can slide onto any rod. The puzzle starts with the disks in a neat stack in ascending order of size on one rod, the smallest at the top, thus making a conical shape. The following figure shows the initial state of the Tower of Hanoi with 5 disks.The objective of the puzzle is to move the entire stack to another rod, obeying the following simple rules: 1. Only one disk can be moved at a time. 2. Each move consists of taking the upper disk from one of the stacks and placing it on top of another stack i.e. a disk can only be moved if it is the uppermost disk on a stack. 3. No disk may be placed on top of a smaller disk. Assume that initially all the disks are placed on rod A. Write a non-recursive Python function to print out the steps to move all the disks from rod A to rod C via rod B (Hint: a recursive algorithm can be converted into a non-recursive algorithm using stack). The header of the function is: def HanoiTower(n) Here n represents the number of disks. example: when n = 3, the program will output: A -> C 换行 A -> B 换行 C -> B 换行 A -> C 换行 B -> A 换行 B -> C 换行 A -> C
时间: 2023-02-05 14:50:35 浏览: 62
这是一个使用非递归算法实现的汉诺塔问题的 Python 代码:
```python
def HanoiTower(n):
stack = []
stack.append((n, 'A', 'B', 'C'))
while len(stack) > 0:
n, from_rod, aux_rod, to_rod = stack.pop()
if n == 1:
print(from_rod, "->", to_rod)
else:
stack.append((n-1, aux_rod, from_rod, to_rod))
stack.append((1, from_rod, aux_rod, to_rod))
stack.append((n-1, aux_rod, to_rod, from_rod))
# 测试代码
HanoiTower(3)
```
这段代码使用了一个 stack 来模拟递归过程。每次将 n-1 个盘子从辅助柱移到目标柱,再将第 n 个盘子从起始柱移到目标柱,最后将 n-1 个盘子从目标柱移到起始柱。这样就可以在不使用递归的情况下解决汉诺塔问题了。
这段代码的输出结果应该是这样的:
```
A -> C
A -> B
C -> B
A -> C
B -> A
B -> C
A -> C
```
相关问题
Please write a simply code in python, the requirement is as followed: 1. use the list type describe rod A, B and C 2. to The Tower of Hanoi is a mathematical game or puzzle. It consists of three rods, and a number of disks of different sizes which can slide onto any rod. The puzzle starts with the disks in a neat stack in ascending order of size on one rod, the smallest at the top, thus making a conical shape. The following figure shows the initial state of the Tower of Hanoi with 5 disks.The objective of the puzzle is to move the entire stack to another rod, obeying the following simple rules: 1. Only one disk can be moved at a time. 2. Each move consists of taking the upper disk from one of the stacks and placing it on top of another stack i.e. a disk can only be moved if it is the uppermost disk on a stack. 3. No disk may be placed on top of a smaller disk. Assume that initially all the disks are placed on rod A. Write a non-recursive Python function to print out the steps to move all the disks from rod A to rod C via rod B (Hint: a recursive algorithm can be converted into a non-recursive algorithm using stack). The header of the function is: def HanoiTower(n) Here n represents the number of disks.
首先,我们可以使用 Python 的列表类型来描述棒 A,B 和 C:
```
rod_A = []
rod_B = []
rod_C = []
```
然后,我们可以定义一个非递归的 Python 函数来打印汉诺塔的移动步骤:
```
def HanoiTower(n):
stack = []
stack.append((n, 'A', 'B', 'C'))
while stack:
n, from_rod, aux_rod, to_rod = stack.pop()
if n == 1:
print(f'Move disk from rod {from_rod} to rod {to_rod}')
else:
stack.append((n-1, aux_rod, from_rod, to_rod))
stack.append((1, from_rod, aux_rod, to_rod))
stack.append((n-1, to_rod, aux_rod, from_rod))
# 调用函数
HanoiTower(5)
```
这样,我们就可以使用非递归的方式来解决汉诺塔问题了。
Link library type specifier "debug" is followed by specifier "optimized" instead of a library name. The first specifier will be ignored.
这个错误提示通常出现在使用 Visual Studio 编译 C++ 代码时。它的意思是在链接库的时候,出现了一个不正确的参数顺序。
为了解决这个问题,你需要检查你的项目设置,看看是否有针对同一个库同时设置了“debug”和“optimized”选项。例如,你可能在调试模式下使用了一个带有“debug”标记的库,而在发布模式下使用了一个带有“optimized”标记的库,但是在项目设置中将这两个标记混合使用了。
要解决这个问题,你需要在项目设置中将这些选项正确设置,或者在代码中手动指定正确的库。
相关推荐
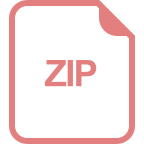
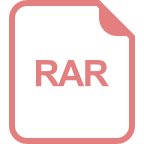
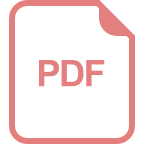












