solovary-stassen素性检验算法C语言
时间: 2023-07-10 22:39:13 浏览: 57
Solovay-Strassen素性检验算法是一种基于欧拉准则的素性检验算法。下面是一个用 C 语言实现的 Solovay-Strassen 素性检验算法的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <math.h>
int modular_exponentiation(int base, int exponent, int modulus)
{
int result = 1;
while (exponent > 0)
{
if (exponent % 2 == 1)
{
result = (result * base) % modulus;
}
base = (base * base) % modulus;
exponent = exponent / 2;
}
return result;
}
int jacobi_symbol(int a, int n)
{
if (n <= 0 || n % 2 == 0)
{
return 0;
}
int j = 1;
if (a < 0)
{
a = -a;
if (n % 4 == 3)
{
j = -j;
}
}
while (a != 0)
{
while (a % 2 == 0)
{
a = a / 2;
if (n % 8 == 3 || n % 8 == 5)
{
j = -j;
}
}
int temp = a;
a = n;
n = temp;
if (a % 4 == 3 && n % 4 == 3)
{
j = -j;
}
a = a % n;
}
if (n == 1)
{
return j;
}
return 0;
}
int is_prime(int n, int k)
{
if (n <= 1 || n == 4)
{
return 0;
}
if (n <= 3)
{
return 1;
}
while (k > 0)
{
int a = 2 + rand() % (n - 3);
int j = jacobi_symbol(a, n);
int x = modular_exponentiation(a, (n - 1) / 2, n);
if (j == 0 || x != j)
{
return 0;
}
k--;
}
return 1;
}
int main()
{
srand(time(NULL));
int n, k;
printf("Enter a number to check if it is prime: ");
scanf("%d", &n);
printf("Enter the number of iterations for the test: ");
scanf("%d", &k);
if (is_prime(n, k))
{
printf("%d is probably prime.\n", n);
}
else
{
printf("%d is composite.\n", n);
}
return 0;
}
```
这个程序中,`modular_exponentiation` 函数用于计算模幂,`jacobi_symbol` 函数用于计算雅可比符号,`is_prime` 函数用于执行素性检验。在 `main` 函数中,用户输入要检验的数和测试的迭代次数,然后调用 `is_prime` 函数进行素性检验。最终输出结果。
相关推荐






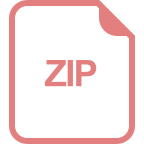
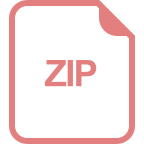
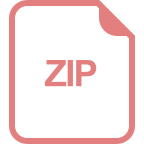