#include<stdio.h> struct Numbers { int nums[20]; int count; int total; }; void findNumbers(struct Numbers *nums) { for (int i = 100;i <= 200;i++){ if(i%6==0 && i%8==0){ nums->nums[nums->count] = i; nums->count++; nums->total +=i; } } } void printNumbers(struct Numbers *nums) { printf("能同时被6和8整除的数有:"); for (int i=0;i<nums->count;i++) { printf(" %d",nums->nums[i]); } printf("\n这些数的和为;%d\n",nums->total); } int main() { struct Numbers nums = {{0},0,0}; findNumbers(&nums); printNumbers(&nums); return 0; }找出主函数并解释它的含义
时间: 2024-04-06 21:28:59 浏览: 43
主函数是程序的入口,它是程序中必须存在的部分。在这段代码中,主函数的名称是`main`,它返回一个整型值,表示程序运行的状态。在主函数中,首先定义了一个名为`nums`的结构体变量,其成员包括一个整型数组`nums`、一个计数器`count`和一个总和`total`,这个结构体用于存储能同时被6和8整除的数的相关信息。
接着,主函数调用`findNumbers`函数,传入指向`nums`结构体的指针,用于查找能同时被6和8整除的数,并将这些数存储到`nums`结构体中。然后,主函数调用`printNumbers`函数,同样传入指向`nums`结构体的指针,用于输出能同时被6和8整除的数以及它们的总和。
最后,主函数返回0,表示程序运行成功。
相关问题
#include<stdio.h> #include<mem.h> #define OK 1 #define ERROR 0 #define MAX_SIZE 100 typedef int Status; typedef struct{ int nums[3]; int id; int operation; int parentId; int level; }ElemType; int cmpArray(int a[], int b[], int n) { int i; for(i=0;i<n;i++) if(a[i] != b[i]) return false; return true; } void BFS(int start[3], int end[3]) { ElemType openTable[MAX_SIZE]; int openFront = 0; int openRear = 0; ElemType CloseTable[MAX_SIZE]; int closeLen=0; bool isSuccess = false; ElemType tmpElem; int count; for(int i=0;i<3;i++) tmpElem.nums[i] = start[i]; // memcpy(tmpElem.nums, start, sizeof(start)); printf("%d %d %d\n", tmpElem.nums[0],tmpElem.nums[1],tmpElem.nums[2]); tmpElem.level = 1; tmpElem.parentId = -1; tmpElem.operation = -1; openTable[openRear] = tmpElem; openRear = (openRear + 1) % MAX_SIZE; while(openRear != openFront) { tmpElem = openTable[openFront]; openFront = (openFront + 1)%MAX_SIZE; printf("[%d %d %d], %c, %d\n", tmpElem.nums[0], tmpElem.nums[1] , tmpElem.nums[2], tmpElem.operation, tmpElem.level ); if(cmpArray(tmpElem.nums, end, 3) && tmpElem.level == 4) { isSuccess = true; break; } else if(tmpElem.level >= 4) { break; } ElemType childElem; for(int i=0;i<3;i++) { if(tmpElem.operation != 'a'+i) { count++; for(int i=0;i<3;i++) childElem.nums[i] = tmpElem.nums[i]; childElem.operation = 'a' + i; // memcpy(childElem.nums, tmpElem.nums, sizeof(tmpElem.nums)); childElem.nums[i] = (childElem.nums[i] + 1) % 2; childElem.parentId = tmpElem.id; childElem.id = count; childElem.level = tmpElem.level + 1; openTable[openRear] = childElem; openRear = (openRear + 1)%MAX_SIZE; } } } if(isSuccess) { printf("success!"); } else { printf("failed"); } } int main() { int a[3]={0,1,0}; int b[3]={1,1,1}; int c[3] = {0,0,0}; BFS(a,b); return 0; } 使用以上程序框架编写C语言代码,解决八数码问题
您可以使用以下代码来解决八数码问题:
```c
#include<stdio.h>
#include<string.h>
#include<stdbool.h>
#define MAX_SIZE 100
typedef struct{
int nums[3][3];
int id;
int operation;
int parentId;
int level;
}ElemType;
void printMatrix(int matrix[3][3]){
for(int i=0;i<3;i++){
for(int j=0;j<3;j++){
printf("%d ", matrix[i][j]);
}
printf("\n");
}
}
bool cmpMatrix(int a[3][3], int b[3][3]){
for(int i=0;i<3;i++){
for(int j=0;j<3;j++){
if(a[i][j] != b[i][j])
return false;
}
}
return true;
}
void BFS(int start[3][3], int end[3][3]){
ElemType openTable[MAX_SIZE];
int openFront = 0;
int openRear = 0;
ElemType CloseTable[MAX_SIZE];
int closeLen=0;
bool isSuccess = false;
ElemType tmpElem;
int count = 0;
memcpy(tmpElem.nums, start, sizeof(start));
printMatrix(tmpElem.nums);
tmpElem.level = 1;
tmpElem.parentId = -1;
tmpElem.operation = -1;
openTable[openRear] = tmpElem;
openRear = (openRear + 1) % MAX_SIZE;
while(openRear != openFront){
tmpElem = openTable[openFront];
openFront = (openFront + 1) % MAX_SIZE;
printMatrix(tmpElem.nums);
printf("Operation: %c, Level: %d\n", tmpElem.operation, tmpElem.level);
if(cmpMatrix(tmpElem.nums, end) && tmpElem.level == 4){
isSuccess = true;
break;
}
else if(tmpElem.level >= 4){
break;
}
ElemType childElem;
for(int i=0;i<3;i++){
for(int j=0;j<3;j++){
if(tmpElem.nums[i][j] == 0){
if(i > 0 && tmpElem.operation != 'd'){ // move up
count++;
memcpy(childElem.nums, tmpElem.nums, sizeof(tmpElem.nums));
childElem.nums[i][j] = childElem.nums[i-1][j];
childElem.nums[i-1][j] = 0;
childElem.operation = 'u';
childElem.parentId = tmpElem.id;
childElem.id = count;
childElem.level = tmpElem.level + 1;
openTable[openRear] = childElem;
openRear = (openRear + 1) % MAX_SIZE;
}
if(i < 2 && tmpElem.operation != 'u'){ // move down
count++;
memcpy(childElem.nums, tmpElem.nums, sizeof(tmpElem.nums));
childElem.nums[i][j] = childElem.nums[i+1][j];
childElem.nums[i+1][j] = 0;
childElem.operation = 'd';
childElem.parentId = tmpElem.id;
childElem.id = count;
childElem.level = tmpElem.level + 1;
openTable[openRear] = childElem;
openRear = (openRear + 1) % MAX_SIZE;
}
if(j > 0 && tmpElem.operation != 'r'){ // move left
count++;
memcpy(childElem.nums, tmpElem.nums, sizeof(tmpElem.nums));
childElem.nums[i][j] = childElem.nums[i][j-1];
childElem.nums[i][j-1] = 0;
childElem.operation = 'l';
childElem.parentId = tmpElem.id;
childElem.id = count;
childElem.level = tmpElem.level + 1;
openTable[openRear] = childElem;
openRear = (openRear + 1) % MAX_SIZE;
}
if(j < 2 && tmpElem.operation != 'l'){ // move right
count++;
memcpy(childElem.nums, tmpElem.nums, sizeof(tmpElem.nums));
childElem.nums[i][j] = childElem.nums[i][j+1];
childElem.nums[i][j+1] = 0;
childElem.operation = 'r';
childElem.parentId = tmpElem.id;
childElem.id = count;
childElem.level = tmpElem.level + 1;
openTable[openRear] = childElem;
openRear = (openRear + 1) % MAX_SIZE;
}
}
}
}
}
if(isSuccess){
printf("Success!\n");
}
else{
printf("Failed\n");
}
}
int main(){
int start[3][3] = {
{0, 1, 2},
{3, 4, 5},
{6, 7, 8}
};
int end[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 0}
};
BFS(start, end);
return 0;
}
```
在上述代码中,`start`和`end`分别表示初始状态和目标状态的矩阵。`BFS()`函数使用广度优先搜索算法来解决八数码问题。在该函数中,我们使用了一个队列来存储待扩展的节点,以及一个数组来存储已扩展的节点。通过遍历每个节点的相邻节点,并将其加入队列中,直到找到目标状态或达到最大搜索深度。如果找到目标状态,则输出"Success!",否则输出"Failed"。
写一个c语言代码,实现在200个int类型的数中找到重复最多的数
为了在 C 语言中找出200个 int 类型数值数组中重复最多的数字,我们可以采用哈希表的方式来存储每个数字及其出现次数。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define MAX_NUMS 200
#define TABLE_SIZE 1000 // 假设最多有1000种不同的数
typedef struct {
int key;
int count;
} HashEntry;
// 使用链表作为哈希表的值,因为哈希冲突的概率较低
typedef struct {
HashEntry entry;
struct HashNode* next;
} HashNode;
HashNode* hashTable[TABLE_SIZE]; // 初始化哈希表
void insert(int num) {
int index = abs(num) % TABLE_SIZE; // 对数取模得到哈希地址
HashNode* node = &hashTable[index];
if (node->entry.key == num && node->entry.count > 1) {
node->entry.count++;
} else if (node->entry.key != num) {
node->entry.key = num;
node->entry.count = 1;
}
}
int findMostFrequent() {
int max_count = 0;
int most_frequent_num = -1;
for (int i = 0; i < TABLE_SIZE; i++) {
if (hashTable[i].entry.key != 0 && hashTable[i].entry.count > max_count) {
max_count = hashTable[i].entry.count;
most_frequent_num = hashTable[i].entry.key;
}
}
return most_frequent_num;
}
int main() {
int nums[MAX_NUMS];
int n;
printf("请输入数字的数量: ");
scanf("%d", &n);
if (n > MAX_NUMS) {
printf("输入数量超过限制,无法处理。\n");
return 1;
}
printf("请输入%d个整数: ", n);
for (int i = 0; i < n; i++) {
scanf("%d", &nums[i]);
insert(nums[i]);
}
int most_common = findMostFrequent();
if (most_common == -1) {
printf("没有重复的数字。\n");
} else {
printf("重复次数最多的数字是: %d\n", most_common);
}
return 0;
}
```
这个程序首先定义了一个哈希表结构,并使用插入函数 `insert()` 来记录每个数字及其出现的次数。`findMostFrequent()` 函数遍历哈希表,找出出现次数最多的数字。注意,这里假设输入的数字范围不大,不会超过 `TABLE_SIZE`。
阅读全文
相关推荐
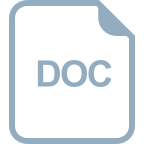
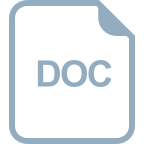
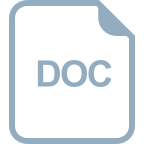













