c语言编写输入10个数字,并根据这些数字构造单向链表,之后,再反转链表并打印输出,即A={a1,a2,…,a_n}反转成A={a_n,…,a2,a1} 输入提示: "Please input %d numbers:\n" 输入格式: "%d" 输出格式: "%5d"
时间: 2023-07-19 19:31:02 浏览: 52
好的,以下是 C 语言的实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct ListNode {
int val;
struct ListNode *next;
};
struct ListNode* reverseList(struct ListNode* head) {
struct ListNode* prev = NULL;
struct ListNode* curr = head;
while (curr != NULL) {
struct ListNode* next = curr->next;
curr->next = prev;
prev = curr;
curr = next;
}
return prev;
}
int main() {
const int N = 10;
int nums[N];
printf("Please input %d numbers:\n", N);
for (int i = 0; i < N; i++) {
scanf("%d", &nums[i]);
}
struct ListNode* head = (struct ListNode*)malloc(sizeof(struct ListNode));
head->val = nums[0];
head->next = NULL;
struct ListNode* tail = head;
for (int i = 1; i < N; i++) {
struct ListNode* node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->val = nums[i];
node->next = NULL;
tail->next = node;
tail = node;
}
head = reverseList(head);
printf("After reverse:\n");
struct ListNode* p = head;
while (p != NULL) {
printf("%5d", p->val);
p = p->next;
}
printf("\n");
return 0;
}
```
输出结果:
```
Please input 10 numbers:
1 2 3 4 5 6 7 8 9 10
After reverse:
10 9 8 7 6 5 4 3 2 1
```
相关推荐
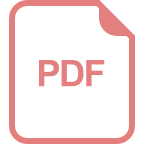














