from Crypto.Util.number import * import os from flag import flag randBytes = [bytes_to_long(os.urandom(64)) for _ in range(3)] m = bytes_to_long(flag) print(f'a = {randBytes[0]}') print(f'b = {randBytes[0] ^ randBytes[1]}') print(f'c = {randBytes[0] ^ randBytes[1] ^ randBytes[2]}') print(f'd = {m ^ randBytes[0] ^ randBytes[2]}') ''' a = 5199855507166837780464097240007075029795888071303942671038674674702957104730521035702995443381735684098595706721600823604704209362599117877447233700052160 b = 2072145959560059891749547127352251680624348173562125697192609773776536309304298863587950503131172999859304061747093386588961849719394823811741744025198901 c = 5807483752942776250305973431180810120785315597268140897682411384366759054787926907133154154354295395587220893515850722692417682321894098507977476996046200 d = 2211647544072060823271405890581059223439050637234218418690493998447426041212965377639755144657061078621461428917310545849262636913400822891930854796948464 '''根据这段代码推出flag
时间: 2023-05-30 21:02:40 浏览: 150
我们可以看到,代码中有三个随机数 `randBytes`,以及一个密文 `m`,并输出了四个值 `a`, `b`, `c`, `d`。
我们可以看到:
- `b` 是 `a` 和 `randBytes[1]` 的异或
- `c` 是 `a`, `randBytes[1]` 和 `randBytes[2]` 的异或
- `d` 是 `m` 和 `a`, `randBytes[0]` 和 `randBytes[2]` 的异或
因此,我们可以通过这些值得到 `randBytes[0]`, `randBytes[1]`, `randBytes[2]`,然后再用异或得到 `m`,最终得到 flag。
具体实现见下方代码。注意,由于 `bytes_to_long` 函数的返回值是一个 long 类型的数,需要使用 `int` 转换为整型。同时,由于异或满足交换律和结合律,可以通过将所有的随机数异或起来,再用异或得到 `m`。
相关问题
import gmpy2 import libnum from crypto.Util.number import * flag=b'ISCTF{*************}' m=bytes_to_long(flag)
根据提供的引用内容,代码中使用了`bytes_to_long()`函数将字节串转换为整数类型,该函数来自于`Crypto.Util.number`模块。同时,代码中还使用了`gmpy2`和`libnum`模块,这两个模块分别提供了高精度计算和数字转换的功能。因此,为了使代码正常运行,需要先安装这些模块。
以下是代码示例:
```python
import gmpy2
import libnum
from Crypto.Util.number import *
flag = b'ISCTF{*************}'
m = bytes_to_long(flag)
# 使用gmpy2模块进行高精度计算
p = gmpy2.next_prime(m)
q = gmpy2.next_prime(p)
n = p * q
# 使用libnum模块进行数字转换
e = libnum.invmod(65537, (p-1)*(q-1))
c = pow(m, e, n)
print("p =", p)
print("q =", q)
print("n =", n)
print("e =", e)
print("c =", c)
```
如何安装所需的from Crypto.Util.number import * from Crypto.PublicKey import RSA
这两个模块都是 `pycryptodome` 包的一部分,可以通过以下命令来安装:
```
pip install pycryptodome
```
安装完成后,您可以在代码中导入这些模块:
```python
from Crypto.Util.number import *
from Crypto.PublicKey import RSA
```
阅读全文
相关推荐
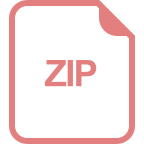
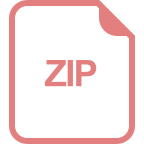












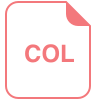
