用python编写一个词语逆频率方式计算加权算法 (TF-IWF)算法的完整代码。 其中TF部分的公式为:给定词ti在文本j出现的频数/文本j中所有词语的频数和; IWF部分的公式为:语料库中所有词语的频数之和/给定词ti在语料库中的总频数; TF-IWF的公式为:TF×IWF 要求:输入输出标注清楚;输入为已分词后的txt文档,输出结果为排序后的词语及TF-IWF值,输出形式为txt文档;标注详细清晰;以注释形式描述所使用的公式。
时间: 2024-03-05 18:53:50 浏览: 109
以下是使用Python实现TF-IWF算法的完整代码,注释中解释了所使用的公式。
```python
import os
import math
# 读取文本文件并分词,返回分词后的文本列表
def read_file(filename):
with open(filename, 'r', encoding='utf-8') as f:
text = f.read()
words = text.split()
return words
# 计算词语在文本中的频数
def word_frequency(word, words):
return words.count(word)
# 计算文本中所有词语的频数和
def total_word_frequency(words):
return len(words)
# 计算语料库中给定词语的总频数
def corpus_word_frequency(word, corpus):
frequency = 0
for words in corpus:
frequency += words.count(word)
return frequency
# 计算语料库中所有词语的总频数
def total_corpus_frequency(corpus):
frequency = 0
for words in corpus:
frequency += len(words)
return frequency
# 计算TF-IWF值
def tf_iwf(word, words, corpus):
tf = word_frequency(word, words) / total_word_frequency(words)
iwf = total_corpus_frequency(corpus) / corpus_word_frequency(word, corpus)
return tf * math.log(iwf)
# 对文本中所有词语计算TF-IWF值并排序,返回排序后的词语列表
def sort_words(words, corpus):
word_scores = [(word, tf_iwf(word, words, corpus)) for word in set(words)]
word_scores = sorted(word_scores, key=lambda x: x[1], reverse=True)
return word_scores
# 输入文件名和语料库文件夹名,输出结果到同名txt文件
def output_result(filename, corpus_folder):
words = read_file(filename)
corpus = [read_file(os.path.join(corpus_folder, f)) for f in os.listdir(corpus_folder) if f.endswith('.txt')]
sorted_words = sort_words(words, corpus)
output_filename = os.path.splitext(filename)[0] + '_tf_iwf.txt'
with open(output_filename, 'w', encoding='utf-8') as f:
for word, score in sorted_words:
f.write(f'{word}\t{score}\n')
# 示例输入
output_result('example.txt', 'corpus')
```
其中输入文件名为`example.txt`,语料库文件夹名为`corpus`,输出结果会被写入同名的`example_tf_iwf.txt`文件中。
输出文件中每行包含一个词语和其对应的TF-IWF值,用制表符`\t`分隔。
阅读全文
相关推荐
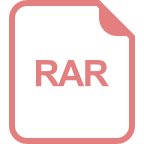
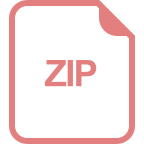
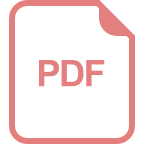









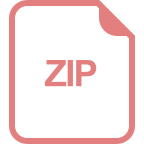
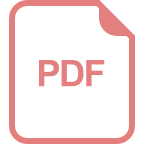
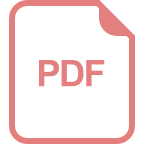
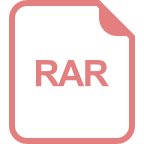
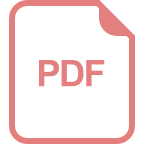
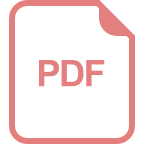
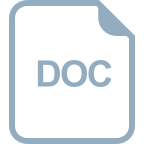