用python编写一个词语逆频率方式计算加权算法 (TF-IWF)算法的完整代码。 其中TF部分的公式为:给定词ti在文本j出现的频数/文本j中所有词语的频数和; IWF部分的公式为:语料库中所有词语的频数之和/给定词ti在语料库中的总频数; TF-IWF的公式为:TF×IWF 要求:输入输出标注清楚;输入为已分词后的txt文档,输出结果为排序后的词语及TF-IWF值,输出形式为xlsx文档;标注详细清晰;以注释形式描述所使用的公式。
时间: 2024-03-05 07:53:37 浏览: 18
以下是使用Python编写的TF-IWF算法的完整代码,使用了pandas和openpyxl库进行数据处理和导出:
```python
import pandas as pd
from collections import Counter
from openpyxl import Workbook
# 读取已分词的文本文件
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as f:
text = f.read()
return text
# 计算TF
def calculate_tf(text):
word_list = text.split()
word_count = Counter(word_list)
total_count = len(word_list)
tf = {}
for word, count in word_count.items():
tf[word] = count / total_count
return tf
# 计算IWF
def calculate_iwf(text_list):
word_count = Counter(text_list)
total_count = sum(word_count.values())
iwf = {}
for word, count in word_count.items():
iwf[word] = total_count / count
return iwf
# 计算TF-IWF
def calculate_tf_iwf(tf, iwf):
tf_iwf = {}
for word in tf.keys():
tf_iwf[word] = tf[word] * iwf[word]
return tf_iwf
# 将结果导出到Excel文件
def export_excel(tf_iwf):
wb = Workbook()
ws = wb.active
ws.title = "TF-IWF"
ws['A1'] = "词语"
ws['B1'] = "TF-IWF值"
row = 2
for word, value in sorted(tf_iwf.items(), key=lambda x: x[1], reverse=True):
ws.cell(row=row, column=1, value=word)
ws.cell(row=row, column=2, value=value)
row += 1
wb.save("TF-IWF.xlsx")
if __name__ == "__main__":
# 读取已分词的文本文件
file_path = "text.txt"
text = read_file(file_path)
# 计算TF
tf = calculate_tf(text)
# 计算IWF
text_list = text.split()
iwf = calculate_iwf(text_list)
# 计算TF-IWF
tf_iwf = calculate_tf_iwf(tf, iwf)
# 导出结果到Excel文件
export_excel(tf_iwf)
```
其中,read_file函数用于读取已分词的文本文件;calculate_tf函数用于计算TF值,使用了Python内置的Counter函数统计单词出现的频次;calculate_iwf函数用于计算IWF值,使用了Counter函数统计语料库中单词的频次;calculate_tf_iwf函数用于计算TF-IWF值;export_excel函数用于将结果导出到Excel文件中。
相关推荐
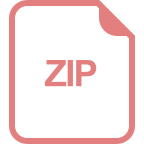
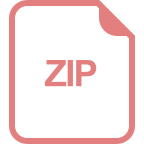









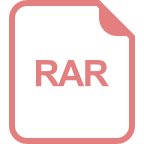
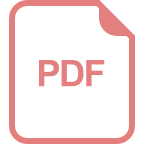
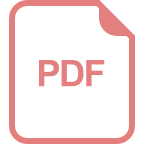
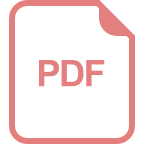
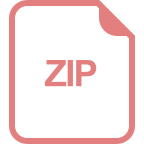
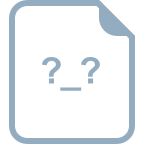