写一个pid控制温度的c代码,温度要求不断变化
时间: 2024-02-18 21:37:00 浏览: 55
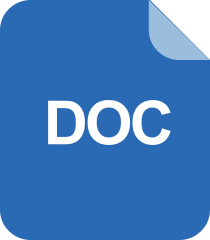
温度控制的PID算法的C语言程序
以下是一个基本的PID控制温度的C代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define TARGET_TEMP 50.0 // 目标温度
#define KP 2.0 // 比例系数
#define KI 0.5 // 积分系数
#define KD 0.1 // 微分系数
double pid_control(double current_temp, double last_error, double integral) {
double error = TARGET_TEMP - current_temp; // 计算当前误差
double derivative = error - last_error; // 计算微分项
integral += error; // 计算积分项
double output = KP * error + KI * integral + KD * derivative; // 计算控制输出
return output;
}
int main() {
srand(time(NULL));
double current_temp = 25.0; // 当前温度
double last_error = 0.0; // 上一次误差
double integral = 0.0; // 积分项
while (1) {
double target_temp = (double)rand() / RAND_MAX * 100.0; // 模拟目标温度的变化
double output = pid_control(current_temp, last_error, integral); // 计算控制输出
current_temp += output; // 更新当前温度
printf("Current temperature: %.2f, Target temperature: %.2f\n", current_temp, target_temp);
if (current_temp >= target_temp - 0.1 && current_temp <= target_temp + 0.1) {
printf("Target temperature reached.\n");
break;
}
last_error = TARGET_TEMP - current_temp; // 保存当前误差
integral = 0.0; // 清空积分项
usleep(1000000); // 等待1秒
}
return 0;
}
```
该代码模拟了目标温度的不断变化,通过PID控制算法来调整当前温度以尽可能接近目标温度。其中,KP、KI和KD分别是比例、积分和微分系数,需要根据实际情况进行调整。
阅读全文
相关推荐
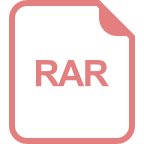
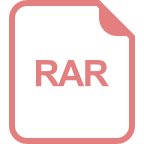
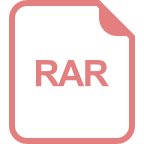
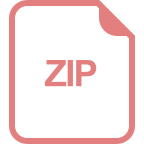
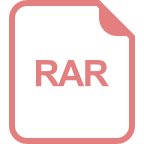
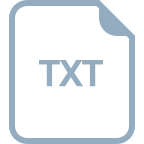
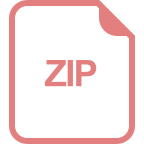
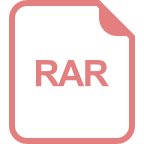
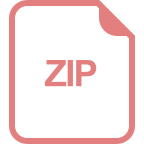
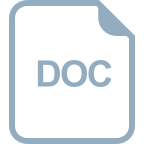





