MATLAB实现基于传统k-means算法在成绩分析的应用代码
时间: 2024-02-11 08:06:45 浏览: 90
以下是MATLAB实现基于传统k-means算法在成绩分析的应用代码示例:
```matlab
% Load data
data = load('grades_data.mat');
X = data.X;
% Initialize k-means parameters
K = 3; % number of clusters
max_iters = 10; % maximum number of iterations
initial_centroids = kMeansInitCentroids(X, K);
% Run k-means algorithm
[centroids, idx] = runkMeans(X, initial_centroids, max_iters);
% Visualize results
plotDataPoints(X, idx, K);
title('K-Means Clustering Results');
% Compute and display cluster centroids
fprintf('Cluster centroids:\n');
fprintf(' %f %f\n', centroids');
% Functions for k-means algorithm
function [centroids, idx] = runkMeans(X, initial_centroids, max_iters)
%RUNKMEANS runs the K-Means algorithm on data matrix X, where each row of X
%is a single example
% Initialize values
[m, n] = size(X);
K = size(initial_centroids, 1);
centroids = initial_centroids;
idx = zeros(m, 1);
% Run K-Means
for i=1:max_iters
% For each example in X, assign it to the closest centroid
idx = findClosestCentroids(X, centroids);
% Given the memberships, compute new centroids
centroids = computeCentroids(X, idx, K);
end
end
function centroids = computeCentroids(X, idx, K)
%COMPUTECENTROIDS returns the new centroids by computing the means of the
%data points assigned to each centroid.
[m, n] = size(X);
centroids = zeros(K, n);
for i=1:K
% Find all examples assigned to centroid i
examples = X(idx == i, :);
% Compute new centroid location as mean of examples
if ~isempty(examples)
centroids(i, :) = mean(examples);
end
end
end
function idx = findClosestCentroids(X, centroids)
%FINDCLOSESTCENTROIDS computes the centroid memberships for every example
%in X where each row in X is a single example.
K = size(centroids, 1);
idx = zeros(size(X,1), 1);
% For each example in X, find closest centroid
for i=1:size(X,1)
distances = zeros(K, 1);
for j=1:K
distances(j) = sum((X(i,:) - centroids(j,:)) .^ 2);
end
[~, idx(i)] = min(distances);
end
end
function centroids = kMeansInitCentroids(X, K)
%KMEANSINITCENTROIDS This function initializes K centroids that are to be
%used in K-Means on the dataset X
% Initialize centroids to random examples
centroids = X(randperm(size(X,1), K), :);
end
function plotDataPoints(X, idx, K)
%PLOTDATAPOINTS plots data points in X, coloring them so that those with
%the same index assignments in idx have the same color
% Create palette
palette = hsv(K + 1);
colors = palette(idx, :);
% Plot data points
scatter(X(:,1), X(:,2), 15, colors);
end
```
这里使用了一个成绩数据集 `grades_data.mat`,其中包含了每个学生的数学和语文成绩。你可以根据自己的数据集进行修改。
阅读全文
相关推荐
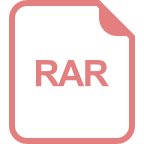
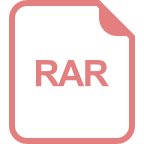
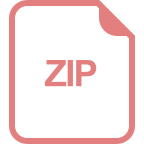
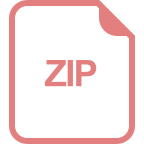
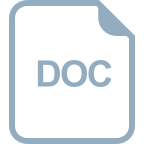
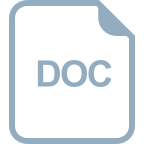
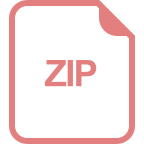
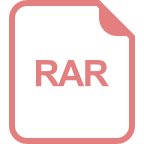
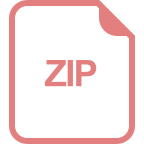
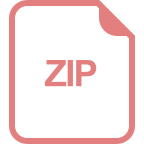
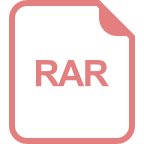
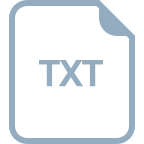
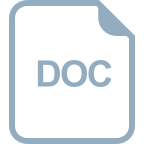
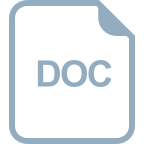
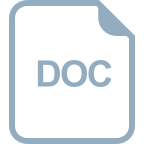