c++ dlib 19.24 怎么处理模糊的人脸
时间: 2024-02-07 20:01:47 浏览: 30
处理模糊的人脸是一个比较复杂的问题,需要结合多种方法来提高识别的准确性。以下是一些处理模糊人脸的方法:
1. 图像增强:通过增强图像的对比度、亮度等参数,可以使图像更加清晰,从而提高人脸识别的准确性。
2. 多模型融合:使用多个不同的人脸识别模型,将它们的结果进行融合,可以提高人脸识别的准确性。
3. 模糊图像去模糊:使用图像去模糊算法对模糊的人脸图像进行处理,可以提高人脸识别的准确性。比如可以尝试使用Dlib库提供的基于Laplacian金字塔的图像去模糊算法。
4. 数据增强:使用更多的数据集进行训练,可以提高人脸识别的准确性。同时,数据增强技术也可以用来增强训练数据集,从而提高识别准确性。
需要注意的是,处理模糊的人脸是一个比较复杂的问题,可能需要结合多种方法来提高识别的准确性。同时,每种方法都有自己的优缺点,需要根据实际情况进行选择。
相关问题
dlib 19.24 c++ 人脸矫正的例子
以下是使用dlib 19.24 c++进行人脸矫正的示例代码:
```c++
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/image_processing.h>
#include <dlib/gui_widgets.h>
#include <dlib/image_io.h>
using namespace dlib;
int main()
{
try
{
// Load the face detection model.
frontal_face_detector detector = get_frontal_face_detector();
shape_predictor sp;
deserialize("shape_predictor_68_face_landmarks.dat") >> sp;
// Load the image.
array2d<rgb_pixel> img;
load_image(img, "test.jpg");
// Detect faces.
std::vector<rectangle> dets = detector(img);
// Find the pose of each face.
std::vector<full_object_detection> shapes;
for (unsigned long i = 0; i < dets.size(); ++i)
shapes.push_back(sp(img, dets[i]));
// Draw the detected faces.
image_window win;
win.set_image(img);
win.add_overlay(dets, rgb_pixel(255, 0, 0));
// Draw the facial landmarks on each face.
for (unsigned long i = 0; i < shapes.size(); ++i)
win.add_overlay(render_face_detections(shapes[i]));
// Get the affine transform for each face and apply it to the image.
for (unsigned long i = 0; i < shapes.size(); ++i)
{
rectangle rect = shapes[i].get_rect();
full_object_detection shape = shapes[i];
matrix<double, 3, 3> trans = get_affine_transform(shape);
array2d<rgb_pixel> face_chip;
extract_image_chip(img, get_face_chip_details(shape,150,0.25), face_chip);
transform_image(face_chip, face_chip, interpolate_bilinear());
win.add_overlay(image_window::overlay_rect(rect, rgb_pixel(255, 0, 0), "face"));
win.add_overlay(image_window::overlay_rect(get_face_chip_details(shape,150,0.25), rgb_pixel(0, 255, 0), "chip"));
win.add_overlay(render_face_detections(shapes[i], rgb_pixel(0, 255, 0)));
save_png(face_chip, "face_chip_" + std::to_string(i+1) + ".png");
}
// Wait for the user to close the window.
win.wait_until_closed();
}
catch (std::exception& e)
{
std::cout << e.what() << std::endl;
}
}
```
此示例代码使用dlib的面部检测器和形状预测器来检测图像中的面部,并使用面部的关键点来获取每个面部的仿射变换矩阵。然后,它将这些仿射变换应用于原始图像中的面部,以便进行矫正。最后,它将矫正后的面部保存为图像文件。
注意:在使用此示例代码之前,您需要下载dlib的面部检测器和形状预测器,并将其保存在与示例代码相同的目录中。您还需要将示例代码中的“test.jpg”更改为您要矫正的实际图像文件名。
帮我用c++ dlib19.24 写一段人脸矫正的程序
好的,这里是使用C++和Dlib19.24库实现人脸矫正的示例代码,供参考:
```c++
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/image_processing.h>
#include <dlib/gui_widgets.h>
#include <iostream>
using namespace dlib;
using namespace std;
int main()
{
try
{
// 读取输入图像
array2d<unsigned char> img;
load_image(img, "input.jpg");
// 初始化人脸检测器
frontal_face_detector detector = get_frontal_face_detector();
// 使用检测器查找输入图像中的人脸
std::vector<rectangle> dets = detector(img);
// 初始化形状预测器
shape_predictor sp;
deserialize("shape_predictor_68_face_landmarks.dat") >> sp;
// 循环遍历每个检测到的人脸
for (unsigned long j = 0; j < dets.size(); ++j)
{
// 获取人脸的关键点
full_object_detection shape = sp(img, dets[j]);
// 计算人脸的旋转角度
double angle = atan2((shape.part(42).y() + shape.part(39).y() - shape.part(27).y() - shape.part(33).y()), (shape.part(42).x() + shape.part(39).x() - shape.part(27).x() - shape.part(33).x())) * 180 / 3.1415926;
// 计算人脸的中心点
point center((shape.part(42).x() + shape.part(39).x() + shape.part(27).x() + shape.part(33).x()) / 4, (shape.part(42).y() + shape.part(39).y() + shape.part(27).y() + shape.part(33).y()) / 4);
// 构造旋转矩阵
dlib::matrix<double> rotMat(2, 2);
rotMat(0, 0) = cos(angle * 3.1415926 / 180);
rotMat(0, 1) = -sin(angle * 3.1415926 / 180);
rotMat(1, 0) = sin(angle * 3.1415926 / 180);
rotMat(1, 1) = cos(angle * 3.1415926 / 180);
// 构造平移矩阵
dlib::matrix<double, 2, 1> transMat;
transMat(0, 0) = center.x();
transMat(1, 0) = center.y();
// 构造仿射变换矩阵
dlib::matrix<double> affineMat(2, 3);
affineMat = dlib::join_rows(rotMat, transMat);
// 进行仿射变换
dlib::matrix<uint8_t> outputImg(img.nr(), img.nc());
dlib::transform_image(img, outputImg, affineMat, interpolate_bilinear());
// 保存输出图像
save_png(outputImg, "output.png");
}
}
catch (exception& e)
{
cout << e.what() << endl;
}
}
```
这段代码使用Dlib库中的人脸检测器和形状预测器,对输入的图像中的人脸进行矫正,输出矫正后的图像。具体的实现过程包括:
1. 读取输入图像
2. 初始化人脸检测器
3. 使用检测器查找输入图像中的人脸
4. 初始化形状预测器
5. 循环遍历每个检测到的人脸
6. 获取人脸的关键点
7. 计算人脸的旋转角度和中心点
8. 构造旋转矩阵、平移矩阵和仿射变换矩阵
9. 进行仿射变换
10. 保存输出图像
请注意,在使用之前您需要下载并安装Dlib库,并且需要提前下载好shape_predictor_68_face_landmarks.dat文件,该文件可以在Dlib官网上下载。同时,您需要将input.jpg替换成您自己的输入图像路径,将output.png替换成您自己的输出图像路径。
相关推荐
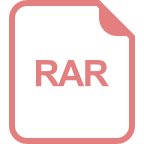
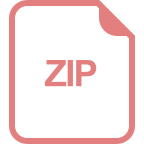










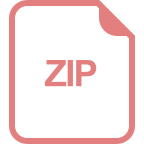