用dlib 19.24 c++帮我写一段做人脸矫正的例子 返回矫正后的 cv::mat
时间: 2024-01-24 17:16:50 浏览: 71
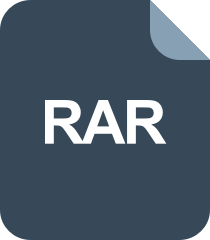
人脸定位实例;c++程序
好的,以下是一个简单的示例代码,使用dlib库中的人脸关键点检测和仿射变换实现人脸矫正:
```c++
#include <dlib/opencv.h>
#include <opencv2/opencv.hpp>
using namespace dlib;
cv::Mat face_alignment(cv::Mat img) {
// Load face detection and landmark detection models
frontal_face_detector detector = get_frontal_face_detector();
shape_predictor sp;
deserialize("shape_predictor_68_face_landmarks.dat") >> sp;
// Convert OpenCV Mat to dlib image
cv_image<bgr_pixel> dlib_img(img);
// Detect faces in the image
std::vector<rectangle> dets = detector(dlib_img);
// Iterate over detected faces
for (rectangle& det : dets) {
// Get the landmarks for the face
full_object_detection shape = sp(dlib_img, det);
// Calculate the transformation matrix for face alignment
std::vector<cv::Point2f> src_points, dst_points;
for (unsigned i = 0; i < shape.num_parts(); ++i) {
const point& pt = shape.part(i);
src_points.push_back(cv::Point2f(pt.x(), pt.y()));
// Define the destination points for face alignment
// You can adjust these points to fit your specific needs
dst_points.push_back(cv::Point2f(0.3*img.cols, 0.3*img.rows));
dst_points.push_back(cv::Point2f(0.7*img.cols, 0.3*img.rows));
dst_points.push_back(cv::Point2f(0.5*img.cols, 0.7*img.rows));
}
cv::Mat H = cv::getAffineTransform(src_points, dst_points);
// Apply the transformation to the original image
cv::Mat aligned_img;
cv::warpAffine(img, aligned_img, H, img.size(), cv::INTER_LINEAR, cv::BORDER_CONSTANT, cv::Scalar());
return aligned_img;
}
return cv::Mat();
}
```
该函数接受一个OpenCV的cv::Mat类型的图像作为输入,并返回矫正后的图像。请注意,该函数加载了一个预训练的人脸关键点检测器和一个形状预测器,这些模型需要从dlib官方网站下载并放置在当前目录下。
该函数做了以下几件事情:
1. 将OpenCV中的cv::Mat图像转换为dlib中的图像类型cv_image<bgr_pixel>;
2. 使用dlib中的人脸检测器检测图像中的人脸,并使用形状预测器获取人脸关键点;
3. 根据关键点计算仿射变换矩阵,将人脸对齐到指定的目标位置;
4. 应用仿射变换将原始图像矫正,并将结果作为cv::Mat类型返回。
在实际使用中,您可以调整目标位置的定义以适应您的具体需求。
阅读全文
相关推荐






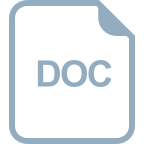
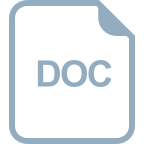
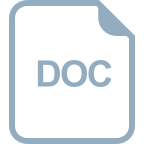






