用dlib 19.24 c++帮我写一段做人脸矫正的例子
时间: 2024-01-22 18:20:45 浏览: 89
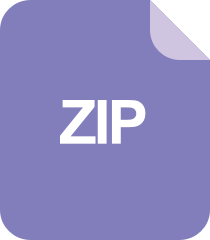
一个使用 dlib 进行人脸识别的例子
好的,请先确保已经安装了dlib库并正确配置了环境变量。
下面是一个简单的人脸矫正的例子:
```c++
#include <iostream>
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/image_processing.h>
#include <dlib/gui_widgets.h>
#include <dlib/image_io.h>
#include <dlib/opencv.h>
#include <opencv2/opencv.hpp>
using namespace dlib;
using namespace std;
int main()
{
try
{
// 加载图像
cv::Mat img = cv::imread("test.jpg");
// 将OpenCV图像转换为dlib图像
cv_image<bgr_pixel> dlib_img(img);
// 初始化人脸检测器
frontal_face_detector detector = get_frontal_face_detector();
// 检测人脸
std::vector<rectangle> dets = detector(dlib_img);
// 初始化人脸关键点检测器
shape_predictor sp;
deserialize("shape_predictor_68_face_landmarks.dat") >> sp;
// 获取人脸关键点
full_object_detection shape = sp(dlib_img, dets[0]);
// 计算人脸矫正矩阵
matrix<double, 3, 3> M = get_rotation_matrix_from_ellipse(shape.get_rect());
// 进行人脸矫正
cv::Mat aligned = cv::Mat(img.size(), img.type());
cv::warpAffine(img, aligned, M, img.size());
// 显示矫正后的图像
cv::imshow("aligned", aligned);
cv::waitKey(0);
}
catch (std::exception& e)
{
cout << e.what() << endl;
}
return 0;
}
```
这里使用了dlib的人脸检测器和人脸关键点检测器,以及提供的人脸矫正函数`get_rotation_matrix_from_ellipse()`,代码中的`shape_predictor_68_face_landmarks.dat`是dlib提供的训练好的模型文件,可以从官网下载。
首先将OpenCV图像转换为dlib图像,然后使用人脸检测器检测出人脸位置,接着使用人脸关键点检测器获取人脸关键点,最后计算人脸矫正矩阵并进行矫正,最终显示矫正后的图像。
注意:这里只处理了检测到的第一个人脸,如果需要处理多张人脸,可以对`dets`进行循环处理。
阅读全文
相关推荐





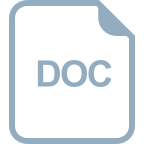
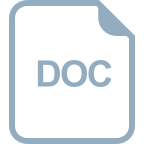
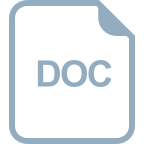
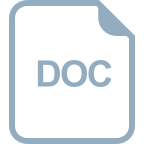






