let point = new ol.geom.Point([item.lon, item.lat]); let feature = new ol.Feature(point); let style = new ol.style.Style({ image: new ol.style.Icon({ className: "my-icon", anchor: [0.5, 0.5], crossOrigin: "anonymous", // 图标缩放比例 scale: 0.8, rotation: item.type == 0 ? (item.heading * Math.PI) / 180 : 0, src: imgUrl, }), }); feature.setStyle(style); let info = { ...item, }; // 设置自定义的信息 feature.setProperties(info); this.circleLayer.getSource().addFeature(feature); 未显示阴影效果
时间: 2024-02-14 15:09:58 浏览: 23
可以尝试给样式对象 `style` 添加 `shadow` 属性,如下所示:
```
let style = new ol.style.Style({
image: new ol.style.Icon({
className: "my-icon",
anchor: [0.5, 0.5],
crossOrigin: "anonymous",
scale: 0.8,
rotation: item.type == 0 ? (item.heading * Math.PI) / 180 : 0,
src: imgUrl,
shadow: new ol.style.Icon({
src: "img/shadow.png",
opacity: 0.5,
scale: 0.8
})
}),
});
```
其中,`shadow` 属性是一个 `ol.style.Icon` 对象,表示图标的阴影效果。在上面的示例中,我们设置了一个 `src` 属性为 `"img/shadow.png"` 的图标作为阴影,并设置了 `opacity` 和 `scale` 两个属性控制阴影的透明度和大小。你可以根据实际情况调整这些参数,使阴影效果更符合你的需求。
相关问题
var wgs84Sphere = new ol.Sphere(6378137);报错 ol.Sphere is not a constructor 请用中文回答 请注意ol.Sphere方法已经 失效了
确实如您所说,OpenLayers 6中的 ol.Sphere 类已经被弃用,因此在新版本中会出现 "ol.Sphere is not a constructor" 的错误。在OpenLayers 6中,您应该使用 `ol/geom/Sphere.js` 模块中的 `getLength()` 和 `getArea()` 方法来计算距离和面积。
以下是示例代码:
```
import Sphere from 'ol/geom/Sphere';
const wgs84Sphere = new Sphere(6378137);
const point1 = [0, 0]; // 经度、纬度,用弧度表示
const point2 = [Math.PI / 2, Math.PI / 2]; // 经度、纬度,用弧度表示
const distance = wgs84Sphere.getLength(point1, point2);
console.log(distance); // 输出结果:10018754.171394622(单位:米)
```
在这个示例中,我们使用 `ol/geom/Sphere.js` 模块中的 `Sphere` 类创建了一个新的 `wgs84Sphere` 实例。然后,我们定义了两个用弧度表示的点(point1 和 point2),并使用 `getLength()` 方法计算它们之间的距离。最后,我们将距离输出到控制台。
com.vividsolutions.jts.geom jar
com.vividsolutions.jts.geom jar是一个用于处理地理空间数据的Java库。该库提供了许多用于几何计算和空间分析的类和方法。
首先,com.vividsolutions.jts.geom jar提供了各种几何对象的表示,如点(Point)、线(Line)、多边形(Polygon)、多点(MultiPoint)、多线(MultiLine)和多边形集合(MultiPolygon)。这些几何对象具有坐标信息和相关的属性,可以用于描述和存储地理空间数据。
其次,该库还提供了许多几何计算和分析的方法。例如,可以使用该库中的方法来计算两个几何对象之间的距离、计算几何对象的面积和长度、判断点是否在几何对象内部等。这些方法可以在地理空间分析和地图应用程序中使用,使得开发者可以轻松处理空间数据的计算和分析任务。
此外,com.vividsolutions.jts.geom jar还支持对几何对象进行空间关系和拓扑分析。开发者可以使用该库中的方法来判断两个几何对象之间的关系,如相等、相交、包含等,或者判断几何对象是否具有拓扑关系,如相邻、相连等。这些方法可以帮助开发者进行复杂的空间数据查询和分析。
总之,com.vividsolutions.jts.geom jar是一个功能强大的Java库,用于处理地理空间数据。它提供了各种几何对象的表示和处理方法,方便开发者进行几何计算、空间分析和拓扑处理。该库在各种地理信息系统和地图应用程序中广泛应用。
相关推荐
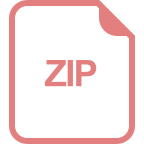













