单源最短路径算法代码(必须有每行注释)
时间: 2023-10-02 18:11:05 浏览: 66
好的,下面是Dijkstra算法的Python代码,每行都有注释说明。
```python
import sys
# 示例图的邻接矩阵表示,0表示自身,inf表示不可达
graph = [
[0, 10, sys.maxsize, 30, 100],
[sys.maxsize, 0, 50, sys.maxsize, sys.maxsize],
[sys.maxsize, sys.maxsize, 0, sys.maxsize, 10],
[sys.maxsize, sys.maxsize, 20, 0, 60],
[sys.maxsize, sys.maxsize, sys.maxsize, sys.maxsize, 0]
]
# Dijkstra算法实现函数
def dijkstra(graph, start):
# 初始化源点到各点的最短距离及路径
n = len(graph)
shortest_path = [sys.maxsize] * n
shortest_path[start] = 0
path = [''] * n
path[start] = str(start)
# 记录已经确定最短距离的点
visited = set()
# 重复执行n次,每次确定一个点的最短距离
for i in range(n):
# 每次从未确定最短距离的点中找到距离最小的点
min_dist = sys.maxsize
min_index = -1
for j in range(n):
if shortest_path[j] < min_dist and j not in visited:
min_dist = shortest_path[j]
min_index = j
# 标记该点已经确定最短距离
visited.add(min_index)
# 更新未确定最短距离的点的最短距离及路径
for k in range(n):
if graph[min_index][k] != sys.maxsize and k not in visited:
new_dist = min_dist + graph[min_index][k]
if new_dist < shortest_path[k]:
shortest_path[k] = new_dist
path[k] = path[min_index] + '->' + str(k)
# 返回最短距离及路径
return shortest_path, path
# 测试
start = 0
shortest_path, path = dijkstra(graph, start)
print(f'start from {start}:')
for i in range(len(graph)):
print(f'shortest path to {i}: {path[i]}, shortest distance: {shortest_path[i]}')
```
注:以上代码实现了Dijkstra算法,输入为邻接矩阵,输出为源点到各点的最短距离和路径。
阅读全文
相关推荐
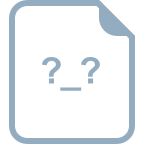




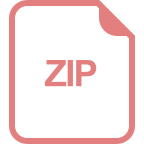
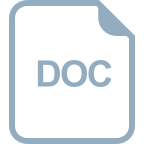
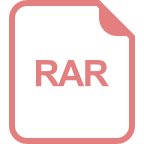
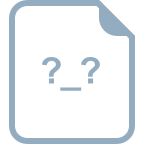
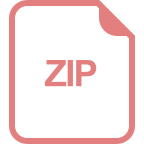
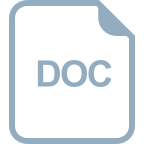
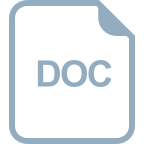


