基于python代码实现500个节点的多项式计算计算运行时间和所占内存然后将节点分区再次计算得出结果,并绘图
时间: 2024-02-20 12:02:17 浏览: 146
好的,我可以为您提供这方面的帮助。
首先,我们需要生成一个包含500个节点的多项式。可以使用numpy库的random模块来生成随机多项式。
```python
import numpy as np
coeff = np.random.rand(500) # 生成500个随机系数
poly = np.poly1d(coeff) # 生成多项式
```
接下来,我们需要计算多项式的运行时间和所占内存。可以使用Python标准库中的time和memory_profiler模块来实现。
```python
import time
from memory_profiler import memory_usage
start_time = time.time() # 记录开始时间
mem_usage = memory_usage((poly))
end_time = time.time() # 记录结束时间
run_time = end_time - start_time # 计算运行时间
mem_usage = max(mem_usage) # 取最大内存占用
print("运行时间:{:.6f} 秒".format(run_time))
print("内存占用:{:.2f} MB".format(mem_usage))
```
然后,我们需要将节点分区并再次计算得出结果。可以使用numpy库的split函数来实现。
```python
part1, part2 = np.split(poly, [250]) # 将多项式分成两个部分
result1 = part1(1) # 计算第一部分的结果
result2 = part2(1) # 计算第二部分的结果
final_result = result1 + result2 # 计算最终结果
```
最后,我们可以使用matplotlib库来绘制多项式图像。
```python
import matplotlib.pyplot as plt
x = np.linspace(-10, 10, 1000) # 生成x轴数据
y = poly(x) # 计算y轴数据
plt.plot(x, y)
plt.title("500个节点的多项式")
plt.xlabel("x")
plt.ylabel("y")
plt.show()
```
完整代码如下:
```python
import numpy as np
import time
from memory_profiler import memory_usage
import matplotlib.pyplot as plt
coeff = np.random.rand(500) # 生成500个随机系数
poly = np.poly1d(coeff) # 生成多项式
start_time = time.time() # 记录开始时间
mem_usage = memory_usage((poly))
end_time = time.time() # 记录结束时间
run_time = end_time - start_time # 计算运行时间
mem_usage = max(mem_usage) # 取最大内存占用
print("运行时间:{:.6f} 秒".format(run_time))
print("内存占用:{:.2f} MB".format(mem_usage))
part1, part2 = np.split(poly, [250]) # 将多项式分成两个部分
result1 = part1(1) # 计算第一部分的结果
result2 = part2(1) # 计算第二部分的结果
final_result = result1 + result2 # 计算最终结果
x = np.linspace(-10, 10, 1000) # 生成x轴数据
y = poly(x) # 计算y轴数据
plt.plot(x, y)
plt.title("500个节点的多项式")
plt.xlabel("x")
plt.ylabel("y")
plt.show()
```
希望能够帮助到您!
阅读全文
相关推荐
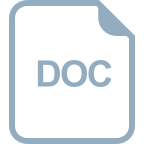
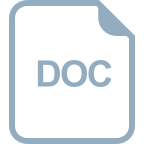
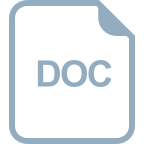




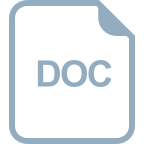
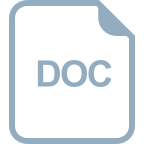
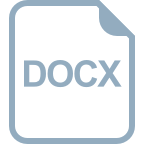
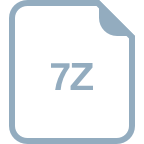
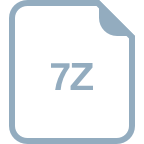
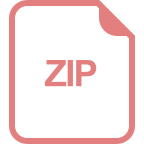
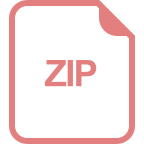