3.先依次输入元素为简单类型(int、char)的数个数据元素建立二叉链表存储的二叉排序树;然后在此二叉排序树上进行元素查找。
时间: 2024-03-04 08:48:50 浏览: 19
好的,您想要构建一个二叉排序树并进行元素查找。我可以为您提供一些示例代码来实现这个功能。以下是一个简单的C++实现:
```c++
#include <iostream>
using namespace std;
// 定义二叉树结构体
struct TreeNode {
int val;
TreeNode *left;
TreeNode *right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
// 向二叉排序树中插入节点
void insert(TreeNode *&root, int val) {
if (root == NULL) {
root = new TreeNode(val);
return;
}
if (val < root->val) {
insert(root->left, val);
} else {
insert(root->right, val);
}
}
// 在二叉排序树中查找元素
bool find(TreeNode *root, int val) {
if (root == NULL) {
return false;
}
if (root->val == val) {
return true;
} else if (val < root->val) {
return find(root->left, val);
} else {
return find(root->right, val);
}
}
int main() {
TreeNode *root = NULL;
int n;
cin >> n; // 输入元素个数
for (int i = 0; i < n; i++) {
int val;
cin >> val; // 输入元素值
insert(root, val); // 将元素插入二叉排序树中
}
int target;
cin >> target; // 输入要查找的元素值
if (find(root, target)) {
cout << "Element " << target << " found in the BST." << endl;
} else {
cout << "Element " << target << " not found in the BST." << endl;
}
return 0;
}
```
在上面的代码中,我们定义了一个二叉树结构体`TreeNode`,并且实现了向二叉排序树中插入节点的函数`insert`以及在二叉排序树中查找元素的函数`find`。在主函数中,我们先输入元素个数,然后依次输入每个元素的值并将它插入二叉排序树中。最后输入要查找的元素值,调用`find`函数进行查找并输出结果。
需要注意的是,我们在`insert`函数和`find`函数中都使用了引用传参,这是因为我们需要修改或访问二叉排序树的根节点。同时,我们在插入节点时使用了递归,这可以简化代码实现。
相关推荐
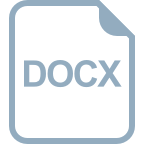
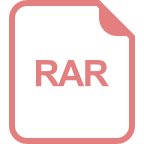
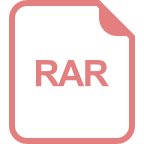














