kotlin file如何转换成kotlin class
时间: 2023-12-22 07:58:44 浏览: 38
要将 Kotlin 文件转换为 Kotlin 类,请按照以下步骤操作:
1. 打开 Kotlin 文件,确保其中只有一个顶级类。如果文件中有多个类,你需要将它们分离成单独的文件。
2. 在顶级类的名称前加上关键字 `class`,这将把顶级类声明为一个 Kotlin 类。例如,如果原始文件中的类名为 `MyClass`,那么新文件的开头应该是 `class MyClass`。
3. 如果顶级类有任何伴生对象或内部类,你需要在新文件中添加适当的嵌套结构。例如,如果原始文件中有一个伴生对象 `CompanionObject`,那么新文件中应该包含 `class MyClass` 和 `companion object CompanionObject` 两个部分。
4. 保存新文件并确保它位于正确的包中。
通过这些步骤,你就可以将 Kotlin 文件转换成 Kotlin 类了。
相关问题
kotlin转java
因为 Kotlin 是在 JVM 上运行的,所以可以轻松地将 Kotlin 代码转换成 Java 代码。
1. 使用 IntelliJ IDEA 进行转换
IntelliJ IDEA 是一个支持 Kotlin 的集成开发环境,可以轻松地将 Kotlin 代码转换成 Java 代码。只需打开 Kotlin 文件,然后选择 "Code" > "Convert Kotlin File to Java",即可将该文件转换为 Java 代码。
2. 使用 Kotlin 工具进行转换
Kotlin 自带一些命令行工具,可以将 Kotlin 代码转换成 Java 代码。在命令行中键入以下命令:
```
kotlinc -include-runtime -d output.jar source.kt
```
然后,在 output.jar 文件所在的目录中运行以下命令:
```
java -jar output.jar
```
这将运行转换后的 Java 代码。
3. 手动转换 Kotlin 代码
可以手动将 Kotlin 代码转换成 Java 代码,但这可能需要花费更多的时间和精力。
例如,以下是一个用 Kotlin 编写的类:
```
data class Person(val name: String, val age: Int)
```
手动转换后,Java 代码如下:
```
public class Person {
private final String name;
private final int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
```
需要注意的是,在 Kotlin 的许多语言构造中,有一些 Java 不支持的特性,例如 Kotlin 的 "when" 表达式和 "let" 函数。在手动转换时,需要考虑这些差异。
kotlin interface
Kotlin 是一种现代的编程语言,它被设计为易于阅读、编写和调试的。Kotlin 的设计理念之一是,它应该能够以一种更自然的方式编写接口。
在 Kotlin 中,接口是一种定义方法签名但不包含实现的语言结构。它们是抽象类的一个弱化版本,更常见于使用契约和标志函数的方式来规定一系列的方法行为。它们也被称为标记接口(marker interfaces)或元接口(meta interfaces)。
Kotlin 中的接口与 Java 语言中的接口类似,但也有些重要的不同点。以下是几个主要的不同点:
1. 实现一个接口是不需要声明一个 `implements` 关键字,而 Java 中则需要声明 `implements` 关键字来指定一个类实现了一个或多个接口。
2. Kotlin 接口中的方法默认都是抽象的,即它们都是 `abstract`。在 Java 中,接口中的方法默认都是 `public` 和 `static` 的,并且可以没有实现。但在 Kotlin 中,所有的方法默认都是 `public` 和 `abstract` 的。
3. Kotlin 允许你在接口中定义属性(字段),这在 Java 中是不可能的。然而,这个特性也可以在对象和结构体中使用,而不仅仅限于接口。
4. Kotlin 中的接口默认会有类型推断。如果一个类实现了一个有默认方法签名的接口,那么它会推断出它的泛型类型。在 Java 中,你不能给默认方法签名签名分配特定的泛型类型,而 Kotlin 则可以做到这一点。
这是一个简单的 Kotlin 接口示例:
```kotlin
// File: Greeting.kt
interface Greeting {
fun sayHello()
}
```
这是一个简单的 Kotlin 类示例,它实现了 `Greeting` 接口:
```kotlin
// File: User.kt
class User {
val name: String = "John"
}
class MainClass : Greeting {
override fun sayHello() {
println("Hello, $name!")
}
}
```
在这个例子中,`User` 类实现了 `Greeting` 接口,并提供了 `sayHello()` 方法的具体实现。`MainClass` 类则继承了 `Greeting` 接口并提供了该方法的具体实现。这样,我们就可以使用 `Greeting` 接口来定义一个契约,并使用具体的类来实现这个契约。
相关推荐
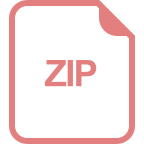
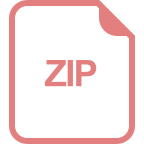
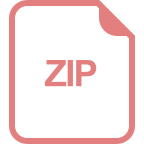












