帮我优化这段代码#include <iostream> #include<iomanip> #include<string> using namespace std; int main() { cout<<"2022116487 叶新于\n"; string message; cout << "请输入要加密的信息:"; getline(cin, message); const string alphabet = "abcdefghijklmnopqrstuvwxyz"; string encrypted_message = ""; for (char c : message) { if (isalpha(c)) { size_t index = alphabet.find(tolower(c)); char new_c = alphabet[(index + alphabet.size() - 5) % alphabet.size()]; encrypted_message += isupper(c) ? toupper(new_c) : new_c; } else { encrypted_message += c; } } cout << "加密后的信息为:" << encrypted_message << endl; system ("pause"); return 0; }
时间: 2023-12-14 15:37:59 浏览: 29
这段代码已经非常简洁了,但还是可以进行一些优化:
1. 可以将输入密码的部分改为使用 cin,避免输入空格时出错:
```
cout << "请输入要加密的信息:";
cin >> ws; // 忽略输入流中的空白字符
getline(cin, message);
```
2. 可以将常量字符串 alphabet 定义为全局变量,避免在循环中重复定义:
```
const string alphabet = "abcdefghijklmnopqrstuvwxyz";
```
3. 可以将加密代码封装成一个函数,提高代码复用性:
```
string encrypt(string message, int shift) {
const string alphabet = "abcdefghijklmnopqrstuvwxyz";
string encrypted_message = "";
for (char c : message) {
if (isalpha(c)) {
size_t index = alphabet.find(tolower(c));
char new_c = alphabet[(index + shift + alphabet.size()) % alphabet.size()];
encrypted_message += isupper(c) ? toupper(new_c) : new_c;
} else {
encrypted_message += c;
}
}
return encrypted_message;
}
```
完整代码如下:
```
#include <iostream>
#include <string>
using namespace std;
const string alphabet = "abcdefghijklmnopqrstuvwxyz";
string encrypt(string message, int shift) {
string encrypted_message = "";
for (char c : message) {
if (isalpha(c)) {
size_t index = alphabet.find(tolower(c));
char new_c = alphabet[(index + shift + alphabet.size()) % alphabet.size()];
encrypted_message += isupper(c) ? toupper(new_c) : new_c;
} else {
encrypted_message += c;
}
}
return encrypted_message;
}
int main() {
cout << "2022116487 叶新于\n";
string message;
cout << "请输入要加密的信息:";
cin >> ws;
getline(cin, message);
cout << "加密后的信息为:" << encrypt(message, -5) << endl;
system("pause");
return 0;
}
```
相关推荐
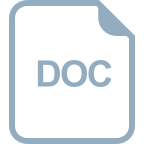
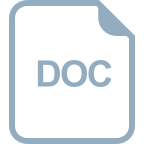
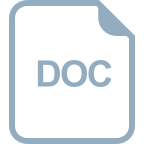















