#include <iostream> #include<iomanip> #include<string> using namespace std; const string alphabet = "abcdefghijklmnopqrstuvwxyz"; string encrypt(string message, int shift) { string encrypted_message = ""; for (char c : message) { if (isalpha(c)) { size_t index = alphabet.find(tolower(c)); char new_c = alphabet[(index + shift + alphabet.size()) % alphabet.size()]; encrypted_message += isupper(c) ? toupper(new_c) : new_c; } else { encrypted_message += c; } } return encrypted_message; } int main() { cout << "2022116487 叶新于\n"; string message; cout << "请输入要加密的信息:"; cin >> ws; getline(cin, message); cout << "加密后的信息为:" << encrypt(message, -5) << endl; system("pause"); return 0; }帮我优化这段代码
时间: 2023-12-14 08:38:09 浏览: 34
以下是对代码的优化建议:
1. 将字符串常量 `alphabet` 声明为 `const char*` 类型,避免不必要的字符串拷贝。
2. 在 `encrypt` 函数中,对于非字母字符,可以直接将其添加到加密后的字符串中,避免不必要的判断和计算。
3. 在 `encrypt` 函数中,可以先将字符转换为小写字母,然后进行加密计算,最后再根据原来的大小写还原加密后的字符,避免重复判断。
4. 在 `main` 函数中,可以使用 `getline` 函数直接读取一行输入,避免 `cin` 函数在读取字符串时留下多余的换行符。
下面是优化后的代码:
```cpp
#include <iostream>
#include <string>
using namespace std;
const char* alphabet = "abcdefghijklmnopqrstuvwxyz";
string encrypt(const string& message, int shift) {
string encrypted_message;
for (char c : message) {
if (isalpha(c)) {
char lc = tolower(c);
size_t index = strchr(alphabet, lc) - alphabet;
char new_c = alphabet[(index + shift + 26) % 26];
encrypted_message += isupper(c) ? toupper(new_c) : new_c;
} else {
encrypted_message += c;
}
}
return encrypted_message;
}
int main() {
cout << "2022116487 叶新于\n";
string message;
cout << "请输入要加密的信息:";
getline(cin, message);
cout << "加密后的信息为:" << encrypt(message, -5) << endl;
system("pause");
return 0;
}
```
相关推荐
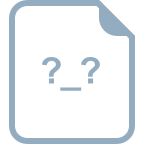















