用分治算法解决包含所有点的最小凸包问题的c语言代码
时间: 2024-03-02 16:53:42 浏览: 69
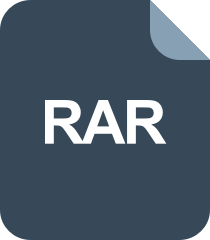
分治法解决凸包问题(C语言实现)

以下是使用分治算法解决包含所有点的最小凸包问题的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_POINTS 1000
typedef struct {
double x;
double y;
} point;
point points[MAX_POINTS];
int n;
int cmp(const void *a, const void *b) {
point *p1 = (point *)a;
point *p2 = (point *)b;
if (p1->x < p2->x) {
return -1;
} else if (p1->x == p2->x) {
if (p1->y < p2->y) {
return -1;
} else if (p1->y == p2->y) {
return 0;
} else {
return 1;
}
} else {
return 1;
}
}
double cross(point p1, point p2, point p3) {
return (p2.x - p1.x) * (p3.y - p1.y) - (p2.y - p1.y) * (p3.x - p1.x);
}
void merge(int l, int m, int r, point *temp) {
int i, j, k;
i = l;
j = m + 1;
k = 0;
while (i <= m && j <= r) {
if (points[i].x < points[j].x) {
temp[k++] = points[i++];
} else {
temp[k++] = points[j++];
}
}
while (i <= m) {
temp[k++] = points[i++];
}
while (j <= r) {
temp[k++] = points[j++];
}
for (i = l, j = 0; i <= r; i++, j++) {
points[i] = temp[j];
}
}
void find_convex_hull(int l, int r, point *hull) {
if (l == r) {
hull[0] = points[l];
return;
}
int m = (l + r) / 2;
point left_hull[MAX_POINTS], right_hull[MAX_POINTS];
find_convex_hull(l, m, left_hull);
find_convex_hull(m + 1, r, right_hull);
int i, j, k;
i = 0;
j = 0;
k = 0;
while (i < m - l + 1 && j < r - m) {
if (left_hull[i].x < right_hull[j].x) {
hull[k++] = left_hull[i++];
} else {
hull[k++] = right_hull[j++];
}
}
while (i < m - l + 1) {
hull[k++] = left_hull[i++];
}
while (j < r - m) {
hull[k++] = right_hull[j++];
}
int nh = k;
for (i = 0; i < nh; i++) {
points[i] = hull[i];
}
k = 1;
for (i = 2; i < nh; i++) {
while (k > 0 && cross(points[k - 1], points[k], points[i]) < 0) {
k--;
}
points[++k] = hull[i];
}
int t = k;
for (i = nh - 2; i >= 0; i--) {
while (k > t && cross(points[k - 1], points[k], hull[i]) < 0) {
k--;
}
points[++k] = hull[i];
}
if (r - l + 1 > n) {
n = r - l + 1;
}
}
int main() {
int i;
point hull[MAX_POINTS];
point temp[MAX_POINTS];
printf("Enter the number of points: ");
scanf("%d", &n);
printf("Enter the points:\n");
for (i = 0; i < n; i++) {
scanf("%lf %lf", &points[i].x, &points[i].y);
}
qsort(points, n, sizeof(point), cmp);
find_convex_hull(0, n - 1, hull);
printf("The minimum convex hull contains %d points:\n", n);
for (i = 0; i < n; i++) {
printf("(%lf, %lf)\n", points[i].x, points[i].y);
}
return 0;
}
```
该代码使用了分治算法来解决包含所有点的最小凸包问题。它首先对所有点按照 x 坐标进行排序,然后使用分治法将点集分为左右两部分,分别对左右两部分求解最小凸包。最后将左右两个凸包合并成一个凸包。
在求解最小凸包的过程中,该算法使用了一个双向扫描的方法来计算凸包的顶点。具体来说,从左到右扫描点集,将凸包的顶点依次加入到凸包中。然后从右到左扫描点集,将凸包的顶点依次加入到凸包中。最后得到的点集就是包含所有点的最小凸包。
阅读全文
相关推荐
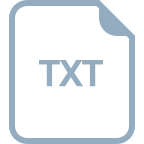

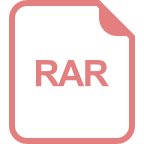
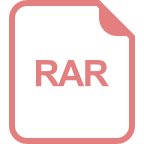
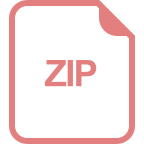
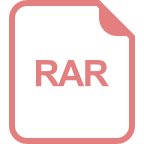
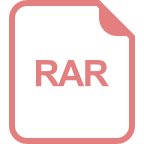
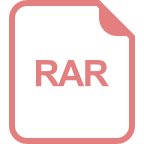
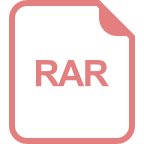
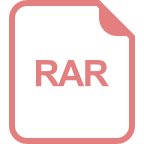
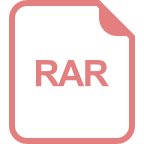
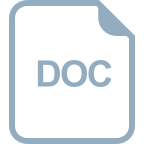
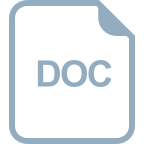
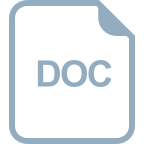
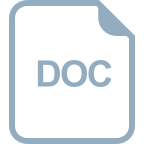