C语言实现:【问题描述】 给定一个带头结点的单链表L和另外一个带头结点的单链表P,P中存储了一些按升序排列的整数。函数printLots(L,P)根据P中指定的位置,打印L中的元素。例如,P=1,3,4,5, 则L中的位于1,3,4和5的元素将被打印出来。实现该函数。 【输入形式】 链表L长度 链表L,以空格区分 链表P长度 链表P,以空格区分 【输出形式】 打印数据,以空格区分 【样例输入】 5 11 22 33 44 55 2 1 3 【样例输出】 11 33
时间: 2024-03-16 18:46:11 浏览: 80
以下是C语言实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结点
struct ListNode {
int val;
struct ListNode *next;
};
// 创建新结点
struct ListNode *createNode(int val) {
struct ListNode *node = (struct ListNode *)malloc(sizeof(struct ListNode));
node->val = val;
node->next = NULL;
return node;
}
// 将新结点插入到链表尾部
void insertTail(struct ListNode **head, int val) {
struct ListNode *node = createNode(val);
if (*head == NULL) {
*head = node;
} else {
struct ListNode *p = *head;
while (p->next != NULL) {
p = p->next;
}
p->next = node;
}
}
// 打印链表
void printList(struct ListNode *head) {
while (head != NULL) {
printf("%d ", head->val);
head = head->next;
}
}
// 释放链表内存
void freeList(struct ListNode **head) {
struct ListNode *p = *head;
while (p != NULL) {
struct ListNode *temp = p;
p = p->next;
free(temp);
}
*head = NULL;
}
// 打印L中P指定位置的元素
void printLots(struct ListNode *L, struct ListNode *P) {
int i = 0;
struct ListNode *p = L;
while (P != NULL) {
int pos = P->val;
while (i < pos && p != NULL) {
p = p->next;
i++;
}
if (p != NULL) {
printf("%d ", p->val);
}
P = P->next;
}
}
int main() {
int lenL, lenP, val;
struct ListNode *headL = NULL, *headP = NULL;
scanf("%d", &lenL);
for (int i = 0; i < lenL; i++) {
scanf("%d", &val);
insertTail(&headL, val);
}
scanf("%d", &lenP);
for (int i = 0; i < lenP; i++) {
scanf("%d", &val);
insertTail(&headP, val);
}
printLots(headL, headP);
freeList(&headL);
freeList(&headP);
return 0;
}
```
输入格式为链表长度和链表元素,通过 `insertTail` 函数将元素插入链表,然后调用 `printLots` 函数打印L中P指定位置的元素,最后释放内存。
阅读全文
相关推荐
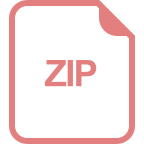
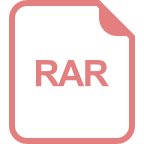
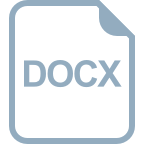















