Cannot cast ufunc 'subtract' output from dtype('float64') to dtype('int32') with casting rule 'same_kind'
时间: 2023-12-13 19:06:11 浏览: 664
这个错误通常是由于尝试将浮点数类型的数组与整数类型的数组进行减法操作导致的。因为浮点数类型与整数类型在Python中有不同的数据类型,所以无法直接进行类型转换。
要解决这个问题,您可以将整数类型的数组转换为浮点数类型的数组,或者在进行减法操作之前进行类型转换。例如,您可以使用以下代码将整数类型的数组转换为浮点数类型的数组:
```python
import numpy as np
int_array = np.array([1, 2, 3])
float_array = int_array.astype(float)
result = float_array - 1.5
```
或者,您可以在进行减法操作之前将浮点数类型的数组转换为整数类型的数组:
```python
import numpy as np
float_array = np.array([1.0, 2.0, 3.0])
int_array = float_array.astype(int)
result = int_array - 1
```
请注意,这些示例代码仅供参考,具体如何更改代码取决于您的具体用例。
相关问题
UFuncTypeError: Cannot cast ufunc 'subtract' output from dtype('float64') to dtype('int64') with casting rule 'same_kind'
这个错误提示意味着你正在尝试将一个浮点数类型的数组和一个整数类型的数组进行减法运算,并且指定了将结果强制转换为整数类型,但是由于浮点数和整数类型的数据范围和精度不同,无法进行这种类型转换。
解决这个问题的方法是:
1. 检查你的代码,确定你的操作是否需要将结果转换为整数类型。如果不是必要的,可以将结果保留为浮点数类型。
2. 如果需要将结果转换为整数类型,可以尝试使用astype()方法将结果强制转换为整数类型,例如:result.astype('int64')。
3. 如果你的操作需要更高精度的计算,可以使用高精度计算库(如NumPy的decimal模块)来进行计算。
请注意,这些解决方案可能需要一些技术知识和资源才能实现。如果需要更具体的帮助,请提供更多的上下文信息,以便我更好地理解你的问题。
numpy.core._exceptions._UFuncOutputCastingError: Cannot cast ufunc 'subtract' output from dtype('float64') to dtype('int32') with casting rule 'same_kind'
The error message indicates that you are trying to perform a subtraction operation in numpy that results in a type casting error. Specifically, you are trying to cast the output of the subtraction operation from a float64 type to an int32 type, which is not allowed with the "same_kind" casting rule.
One way to solve this issue is to explicitly specify the output data type of the operation to be float64 or int32, depending on your needs. Here is an example:
```python
import numpy as np
# create two arrays with different data types
a = np.array([1, 2, 3], dtype=np.int32)
b = np.array([0.5, 1.5, 2.5], dtype=np.float64)
# perform subtraction operation with explicit output data type
c = np.subtract(a, b, dtype=np.float64)
# print result
print(c)
```
In this example, we explicitly specify the output data type of the subtraction operation to be float64 using the `dtype` parameter. This allows the operation to complete without any casting errors.
Alternatively, you can also use the `astype()` method to convert the data type of the array after the operation has completed. For example:
```python
import numpy as np
# create two arrays with different data types
a = np.array([1, 2, 3], dtype=np.int32)
b = np.array([0.5, 1.5, 2.5], dtype=np.float64)
# perform subtraction operation and convert result to int32
c = np.subtract(a, b).astype(np.int32)
# print result
print(c)
```
In this example, we perform the subtraction operation first and then convert the data type of the result to int32 using the `astype()` method. This allows us to perform the operation without any casting errors and then convert the result to the desired data type.
阅读全文
相关推荐
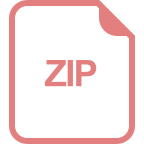
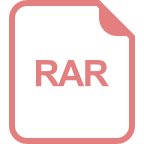
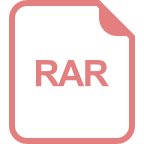






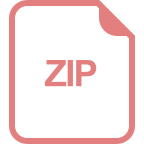
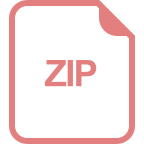
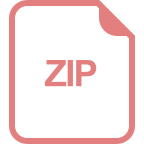
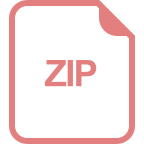