编写详细简洁的C语言程序在 µ’nSP™十六位单片机实验箱 SPLC501 液晶显示器上完成一个简单的按键小游戏,在显示器上随机显示一个10以内的整数,玩家需要快速按下对应次数的按键S20,开发平台为u'nSP IDE,一定需要注意要求是只能用头文件SPLC501User.h,不用其他的头文件,同时需要将之后需要用到的各种变量与函数在前面做一个定义声明 SPLC501 液晶显示模组英文字符显示相关的 API 函数如下: void LCD501_Init(unsigned int InitialData) //初始化液晶显示 void LCD501_ClrScreen(unsigned int Mode) //点亮屏幕或清屏 void LCD501_SetPaintMode(unsigned ModeCode) //设置图形显示模式 unsigned int LCD501_GetPaintMode(void) //获取图形显示模式 void LCD501_FontSet(unsinged int Font) //选择显示字符的字体大小 unsigned int LCD501_FontGet(void) //获得显示字符的字体大小 void LCD501_Char(int x,int y,unsigned int a) //显示字符 void LCD501_PutString(int x,int y,unsigned int a) //显示字符串
时间: 2024-03-14 18:45:05 浏览: 17
以下是一个简单的按键小游戏程序,使用SPLC501User.h头文件,仅使用此头文件中的API函数进行操作:
```
#include "SPLC501User.h"
//声明需要用到的变量和函数
unsigned int score=0; //得分变量
unsigned int target=0; //目标数字变量
unsigned int button_count=0; //按键计数变量
unsigned int button_status=0; //按键状态变量
void delay(unsigned int n) //延时函数
{
unsigned int i;
while(n--)
{
for(i=0;i<1000;i++);
}
}
void update_target(void) //更新目标数字函数
{
target=rand()%10; //生成0~9之间的随机数字
LCD501_ClrScreen(0); //清屏
LCD501_FontSet(0x02); //设置字体大小
LCD501_PutString(0,0,"Target:"); //显示Target:
LCD501_Char(64,0,target+'0'); //显示目标数字
}
void main(void)
{
LCD501_Init(0x00); //初始化液晶显示
update_target(); //更新目标数字
while(1)
{
//显示得分
LCD501_FontSet(0x01); //设置字体大小
LCD501_PutString(0,16,"Score:"); //显示Score:
if(score<10)
{
LCD501_Char(56,16,'0'); //补零
LCD501_Char(64,16,score+'0');
}
else
{
LCD501_Char(56,16,score/10+'0');
LCD501_Char(64,16,score%10+'0');
}
//检测按键状态
if(PINB&0x01) //按键按下
{
if(button_status==0) //按键状态从未按下变为按下
{
button_count++; //按键计数加1
button_status=1; //将按键状态设置为按下
}
}
else //按键未按下
{
button_status=0; //将按键状态设置为未按下
}
//判断游戏是否结束
if(button_count==target) //达成目标
{
score++; //得分加1
update_target(); //更新目标数字
button_count=0; //重置按键计数
}
else if(button_count>target) //超过目标
{
score=0; //得分清零
update_target(); //更新目标数字
button_count=0; //重置按键计数
}
delay(10); //延时10毫秒
}
}
```
该程序使用了一个无限循环来不断更新LCD显示和检测按键状态。在循环中,首先显示得分和目标数字,之后检测按键状态。如果按键被按下,按键计数加1,并将按键状态设置为按下。如果按键未按下,按键状态设置为未按下。之后判断游戏是否结束,如果按键计数达到目标数字,则得分加1,更新目标数字并重置按键计数;如果按键计数超过目标数字,则得分清零,更新目标数字并重置按键计数。最后延时10毫秒。
相关推荐
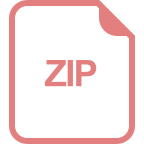
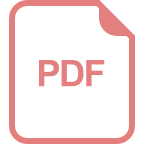














