使用Java定义一个交通工具(Vehicle)类,其具有的属性和方法如下: 属性: 1)速度-speed; 2) 长度-length;3) 宽度-width;4) 高度-height; 方法: 1)移动-move() 2) 加速-speedUp(int num) num: 表示在当前速度上增加的速度值 3) 减速-speedDown(int num) num: 表示在当前速度上减低的速度值 4)对Vehicle类的各个内部字段使用setter/getter 进行封装 5) 创建测试代码,设置输出一个Vehicle对象的各个属性,并测试speedUp()和speedDown()方法。
时间: 2024-02-25 09:53:58 浏览: 56
下面是一个简单的Java代码示例,实现了您要求的交通工具类:
```java
public class Vehicle {
private double speed;
private double length;
private double width;
private double height;
public Vehicle(double speed, double length, double width, double height) {
this.speed = speed;
this.length = length;
this.width = width;
this.height = height;
}
public void move() {
System.out.println("Vehicle is moving");
}
public void speedUp(double num) {
this.speed += num;
System.out.println("Vehicle speed up to " + this.speed);
}
public void speedDown(double num) {
this.speed -= num;
System.out.println("Vehicle speed down to " + this.speed);
}
public double getSpeed() {
return speed;
}
public void setSpeed(double speed) {
this.speed = speed;
}
public double getLength() {
return length;
}
public void setLength(double length) {
this.length = length;
}
public double getWidth() {
return width;
}
public void setWidth(double width) {
this.width = width;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public static void main(String[] args) {
Vehicle vehicle = new Vehicle(50, 5, 2, 2);
System.out.println("Vehicle speed: " + vehicle.getSpeed());
System.out.println("Vehicle length: " + vehicle.getLength());
System.out.println("Vehicle width: " + vehicle.getWidth());
System.out.println("Vehicle height: " + vehicle.getHeight());
vehicle.speedUp(20);
vehicle.speedDown(10);
}
}
```
在上面的代码中,我们定义了一个名为 `Vehicle` 的类,它包含了速度、长度、宽度和高度四个属性,以及移动、加速、减速三个方法,以及各个属性的setter和getter方法。在 `main` 方法中,我们创建了一个 `Vehicle` 对象,并输出了它的各个属性值,然后测试了 `speedUp()` 和 `speedDown()` 方法。
阅读全文
相关推荐
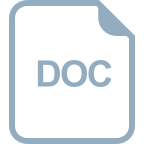
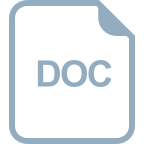
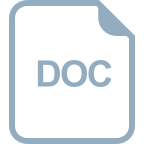




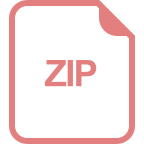










