if(Input.GetMouseButtonUp(0))
时间: 2024-10-21 20:07:28 浏览: 14
`if(Input.GetMouseButtonUp(0))` 是Unity引擎中的一个检查条件,主要用于检测用户是否刚刚释放了鼠标左键(Index 0通常对应于鼠标左键)。这个函数属于`Input`类,`GetMouseButtonUp`方法会返回一个布尔值,如果用户在当前帧松开了鼠标左键,则该值为`true`,否则为`false`。
例如,在游戏场景中,你可以这样使用这个条件:
```csharp
if (Input.GetMouseButtonUp(0))
{
// 如果玩家点击并放开左键,执行相应的操作
RaycastHit hit;
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
if (Physics.Raycast(ray, out hit))
{
// 打开某个UI元素,或者攻击目标...
Debug.Log("Player clicked on " + hit.collider.name);
}
}
```
在这个代码段中,如果玩家点击了屏幕上的某个位置,然后释放鼠标左键,程序将检查是否有碰撞,并根据结果执行相应动作。
相关问题
unity input mousebutton
Unity中的输入系统可以用来检测鼠标按钮的输入。您可以使用以下代码来检测鼠标按钮的按下和释放:
```csharp
void Update()
{
if (Input.GetMouseButtonDown(0))
{
// 左键按下
Debug.Log("Left mouse button pressed");
}
if (Input.GetMouseButtonUp(0))
{
// 左键释放
Debug.Log("Left mouse button released");
}
if (Input.GetMouseButtonDown(1))
{
// 右键按下
Debug.Log("Right mouse button pressed");
}
if (Input.GetMouseButtonUp(1))
{
// 右键释放
Debug.Log("Right mouse button released");
}
// 其他鼠标按钮的检测
// 检测鼠标滚轮滚动
float scroll = Input.mouseScrollDelta.y;
if (scroll != 0f)
{
Debug.Log("Mouse scroll: " + scroll);
}
}
```
在Update()函数中,我们使用`Input.GetMouseButtonDown()`和`Input.GetMouseButtonUp()`来检测鼠标按钮的按下和释放。参数中的数字表示要检测的鼠标按钮,其中0表示左键,1表示右键,2表示中键,依此类推。
同时,我们还可以使用`Input.mouseScrollDelta.y`来检测鼠标滚轮的滚动。返回的值为正数表示向上滚动,负数表示向下滚动。在示例代码中,我们将滚动的值打印到控制台上。
请注意,这段代码应该放在一个继承自MonoBehaviour的脚本中,以便在Unity中运行时调用Update()函数。
请用unity写出,在场景中放置一些3D对象,使用鼠标点击和拖出矩形框选的方法选取场景中的对象。 1.写出点选和圈选的算法说明 2.绘制点选和圈选算法的流程图、 3.在unity中实现题目要求的功能
好的,我会根据你的要求回答你的问题。
1. 点选算法说明:当用户用鼠标点击某个3D对象时,首先需要判断鼠标所在的屏幕位置对应的场景中是否存在3D对象。如果存在,则将该对象选中,否则取消已选中的对象。
圈选算法说明:当用户用鼠标点击并拖拽出一个矩形区域时,需要判断该区域内是否有3D对象。如果存在,则将该对象选中,否则取消已选中的对象。
2. 点选和圈选算法流程图如下:
```
开始
|
|——> 鼠标点击屏幕位置
| |
| |——> 判断是否存在3D对象
| | |
| | |——> 存在3D对象,选中该对象
| | |
| | |——> 不存在3D对象,取消已选中的对象
| |
| |——> 鼠标拖拽出矩形区域
| |
| |——> 判断该区域内是否存在3D对象
| | |
| | |——> 存在3D对象,选中该对象
| | |
| | |——> 不存在3D对象,取消已选中的对象
|
结束
```
3. 在Unity中实现题目要求的功能,可以按照以下步骤进行:
- 在场景中放置一些3D对象。
- 创建一个脚本,并将其附加到摄像机上。
- 在脚本中编写代码,实现点选和圈选算法。
- 使用Raycast判断鼠标点击的位置是否存在3D对象,并选中该对象。
- 使用Physics.OverlapBox检测矩形区域内是否有3D对象,并选中该对象。
- 使用OnGUI绘制矩形框选的效果。
具体实现过程可以参考以下示例代码:
```csharp
using UnityEngine;
public class ObjectSelector : MonoBehaviour
{
private GameObject selectedObject;
private Vector3 mousePosition1;
private Vector3 mousePosition2;
private void Update()
{
// 点选
if (Input.GetMouseButtonDown(0))
{
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
RaycastHit hit;
if (Physics.Raycast(ray, out hit))
{
if (hit.collider.gameObject != selectedObject)
{
if (selectedObject != null)
{
selectedObject.GetComponent<Renderer>().material.color = Color.white;
}
selectedObject = hit.collider.gameObject;
selectedObject.GetComponent<Renderer>().material.color = Color.blue;
}
}
else
{
if (selectedObject != null)
{
selectedObject.GetComponent<Renderer>().material.color = Color.white;
}
selectedObject = null;
}
}
// 圈选
if (Input.GetMouseButtonDown(1))
{
mousePosition1 = Input.mousePosition;
}
if (Input.GetMouseButtonUp(1))
{
mousePosition2 = Input.mousePosition;
Vector3 boxPosition1 = Camera.main.ScreenToWorldPoint(mousePosition1);
Vector3 boxPosition2 = Camera.main.ScreenToWorldPoint(mousePosition2);
Vector3 center = (boxPosition1 + boxPosition2) / 2;
Vector3 size = new Vector3(Mathf.Abs(boxPosition1.x - boxPosition2.x), Mathf.Abs(boxPosition1.y - boxPosition2.y), Mathf.Abs(boxPosition1.z - boxPosition2.z));
Collider[] hitColliders = Physics.OverlapBox(center, size / 2);
foreach (Collider hitCollider in hitColliders)
{
if (hitCollider.gameObject != selectedObject)
{
if (selectedObject != null)
{
selectedObject.GetComponent<Renderer>().material.color = Color.white;
}
selectedObject = hitCollider.gameObject;
selectedObject.GetComponent<Renderer>().material.color = Color.blue;
}
}
}
}
private void OnGUI()
{
if (Input.GetMouseButton(1))
{
Rect rect = new Rect(mousePosition1.x, Screen.height - mousePosition1.y, Input.mousePosition.x - mousePosition1.x, mousePosition1.y - Input.mousePosition.y);
GUI.Box(rect, "");
}
}
}
```
这样,在场景中就可以通过鼠标点击和拖出矩形框选的方法选取对象了。
阅读全文
相关推荐
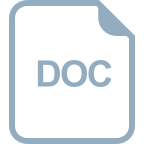
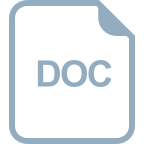
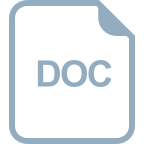
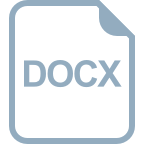
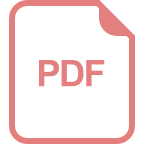
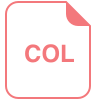



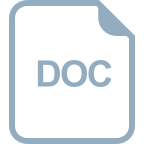
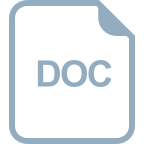
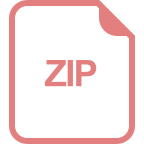
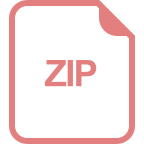
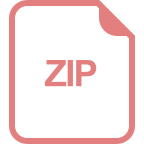