Calculate the magnitude and phase of input frame using IFFT C语言
时间: 2024-03-20 21:39:45 浏览: 101
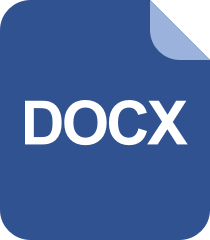
FFT及IFFT的C语言实现
To calculate the magnitude and phase of an input frame using IFFT in C language, you can follow these steps:
1. Define your input frame as an array of complex numbers, where each element is a struct with a real and imaginary component. For example:
```
typedef struct {
float real;
float imag;
} complex;
complex input_frame[N]; // N is the size of your input frame
```
2. Apply the IFFT to your input frame using a function like `ifft()` from a library like FFTW:
```
fftw_plan plan = fftw_plan_dft_1d(N, (fftw_complex*)input_frame, (fftw_complex*)input_frame, FFTW_BACKWARD, FFTW_ESTIMATE);
fftw_execute(plan);
fftw_destroy_plan(plan);
```
This will transform your input frame from the frequency domain to the time domain.
3. Calculate the magnitude and phase of each complex number in the transformed input frame:
```
for (int i = 0; i < N; i++) {
float mag = sqrt(input_frame[i].real * input_frame[i].real + input_frame[i].imag * input_frame[i].imag);
float phase = atan2(input_frame[i].imag, input_frame[i].real);
// do something with mag and phase...
}
```
The magnitude is calculated as the square root of the sum of the squares of the real and imaginary components, and the phase is calculated as the arctangent of the imaginary component divided by the real component.
Note that this is just a basic example, and there are many variations and optimizations you can make depending on your specific application and requirements.
阅读全文
相关推荐


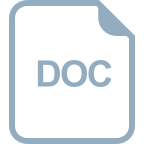
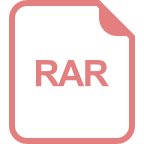
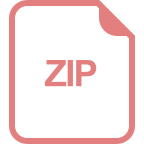
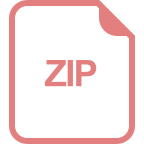
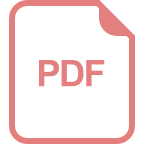
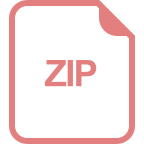
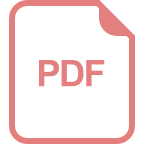
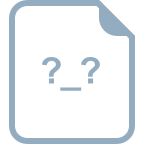
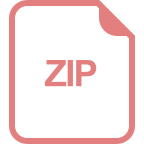
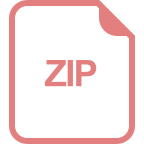
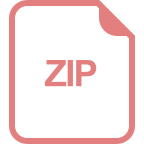
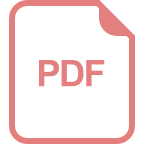
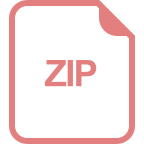
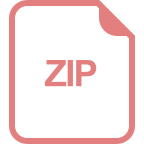