Calculate the output frame using IFFT C语言
时间: 2024-03-19 18:46:30 浏览: 18
要使用C语言计算IFFT输出帧,您需要执行以下步骤:
1. 将输入帧通过FFT转换为频域。
2. 对频域信号进行复数共轭(complex conjugate)操作。
3. 对共轭后的频域信号进行FFT操作。
4. 对FFT输出进行复数共轭操作。
5. 将结果除以信号长度。
6. 对结果进行实部取整(round)操作。
7. 将整数帧作为输出。
以下是一个示例代码,假设FFT结果为cplx[]数组,输出帧为out[]数组,信号长度为N:
```c
#include <math.h>
#include <complex.h>
#define PI 3.14159265358979323846
void ifft(complex double cplx[], double out[], int N) {
int k, n;
complex double sum;
// complex conjugate
for (n = 0; n < N; n++) {
cplx[n] = conj(cplx[n]);
}
// FFT
fft(cplx, N);
// complex conjugate
for (n = 0; n < N; n++) {
cplx[n] = conj(cplx[n]);
}
// divide by N
for (n = 0; n < N; n++) {
cplx[n] /= N;
}
// round and output
for (n = 0; n < N; n++) {
out[n] = round(creal(cplx[n]));
}
}
void fft(complex double cplx[], int N) {
int k, n;
complex double sum, t;
double angle;
for (k = 0; k < N; k++) {
sum = 0;
for (n = 0; n < N; n++) {
angle = 2 * PI * k * n / N;
t = cexp(-I * angle);
sum += cplx[n] * t;
}
cplx[k] = sum;
}
}
```
这是一个非常简单的实现,仅用于说明基本原理。实际上,要正确实现IFFT需要更多的细节和优化。
相关推荐
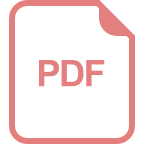














